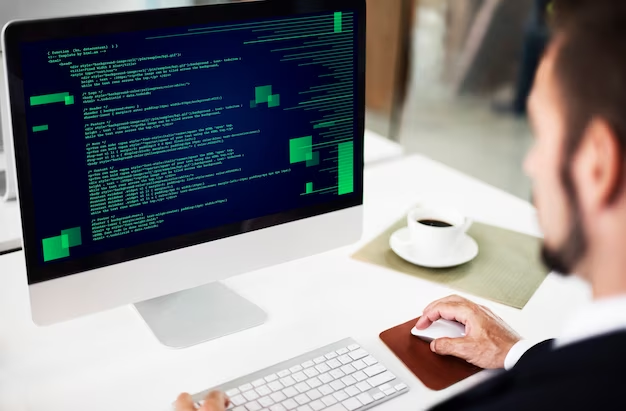
How to Standardize Data in Python
In the world of machine learning, one of the initial steps in feature engineering is data standardization. It’s crucial to ensure that your data is appropriately scaled, especially when dealing with various machine-learning models. Some models, such as linear regression, K-nearest neighbors (KNN), and support vector machines (SVM), are highly sensitive to features with different scales. On the other hand, models like decision trees, bagging, and boosting algorithms typically don’t require data scaling.
The Impact of Data Scaling
The impact of feature scaling on machine learning models is significant. Features with larger value ranges tend to dominate the decision-making process of algorithms because their effects on the outputs are more pronounced. To level the playing field and make sure all features are equally considered during model training, we turn to feature scaling techniques.
Two Popular Feature Scaling Techniques
Two of the most commonly used feature scaling techniques are:
- Z-Score Standardization: Also known as mean normalization, this technique scales the data based on the mean and standard deviation of the dataset;
- Min-Max Normalization: This method scales the data between a specified range, typically 0 and 1.
In this article, we will delve into how to perform z-score standardization of data using Python, specifically utilizing the sklearn and pandas libraries.
Understanding Standardization
In statistics and machine learning, data standardization involves converting data into z-score values, which are based on the mean and standard deviation of the dataset. Essentially, each data point within a feature is transformed into a representative number of standard deviations it deviates from the mean. The result is a dataset with a mean of 0 and values generally ranging between -3 and +3, assuming a normal distribution of data (which holds for approximately 99.9% of data points).
The z-score formula for a given observation x within a feature is as follows:
z = (x – mean) / standard deviation
Let’s consider a simple example to illustrate the concept of standardization.
Standardization Example
Suppose we have a dataset with two features: “Weight” in grams and “Price” in dollars.
Weight (g) | Price ($) |
---|---|
300 | 3 |
250 | 2 |
800 | 5 |
The weights range from 250g to 800g, while prices range from $2 to $5. These different scales make direct comparisons challenging.
Standardizing the Data
We’ll start by standardizing the “Weight” feature:
- Mean of Weight: 450g;
- Standard Deviation of Weight: 248.3277g
For the first observation (Weight = 300g), the z-score is calculated as follows:
z = (300 – 450) / 248.3277 = -0.81
For the second observation (Weight = 250g):
z = (250 – 450) / 248.3277 = -1.064
And for the third observation (Weight = 800g):
z = (800 – 450) / 248.3277 = 1.336
We perform similar calculations for the “Price” feature using its mean and standard deviation.
Standardized Dataset
After standardization, our dataset now looks like this:
Weight (standardized) | Price (standardized) |
---|---|
-0.604 | -0.267 |
-0.805 | -1.069 |
1.409 | 1.336 |
The scales of the features have been aligned, making data visualization and analysis more meaningful.
How to Standardize Data in Python
To perform data standardization in Python, we’ll use the StandardScaler class from the sklearn library. First, let’s create a sample dataset as shown earlier.
import pandas as pd data = {‘Weight (g)’: [300, 250, 800], ‘Price ($)’: [3, 2, 5]} df = pd.DataFrame(data) |
Now, let’s standardize the data:
from sklearn.preprocessing import StandardScaler scaler = StandardScaler()standardized_data = scaler.fit_transform(df) standardized_df = pd.DataFrame(standardized_data, columns=df.columns) |
The standardized_df now contains the standardized data.
Video Explanation
In order to explain this topic in more detail, we have prepared a special video for you. Enjoy watching it!
Advantages of Data Standardization
Standardizing data in machine learning has several advantages that contribute to improved model performance and robustness. Here are some key benefits of data standardization:
1. Improved Model Convergence
When dealing with features of varying scales, machine learning algorithms can take longer to converge or may even fail to converge. Standardizing the data ensures that features are on a common scale, making it easier for optimization algorithms to find the optimal model parameters.
2. Enhanced Model Interpretability
Standardized data simplifies the interpretation of model coefficients. In linear models, for example, the coefficients represent the change in the target variable for a one-unit change in the corresponding feature. With standardized features, these coefficients become more meaningful and directly comparable.
3. Robustness to Outliers
Machine learning models can be sensitive to outliers in the data. Standardization helps mitigate this sensitivity by reducing the impact of extreme values. Features with large scales can dominate the influence of outliers, but after standardization, their influence is limited to a few standard deviations.
4. Better Model Generalization
Standardized data often leads to models that generalize better to unseen data. When features are on a similar scale, the model can make predictions that are more consistent across different subsets of the data, resulting in improved generalization performance.
5. Compatibility with Regularization Techniques
Regularization techniques like L1 and L2 regularization assume that all features have similar scales. Standardization aligns features with these assumptions, allowing regularization to work effectively in controlling model complexity.
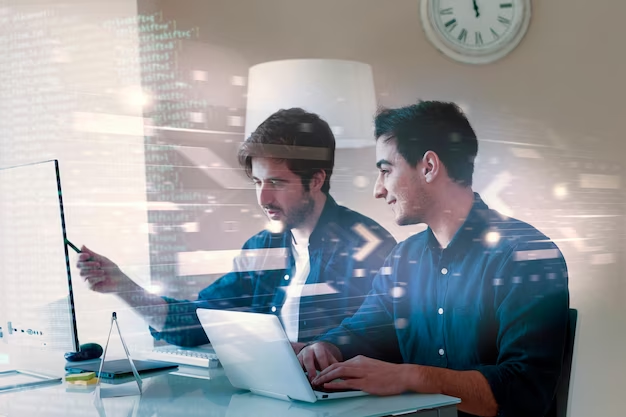
Conclusion
Data standardization is a critical step in preparing your data for machine learning. By scaling features appropriately, you ensure that your models are not biased toward any particular feature due to its scale. In this tutorial, we explored how to standardize data in Python using the z-score standardization technique.
Average Rating