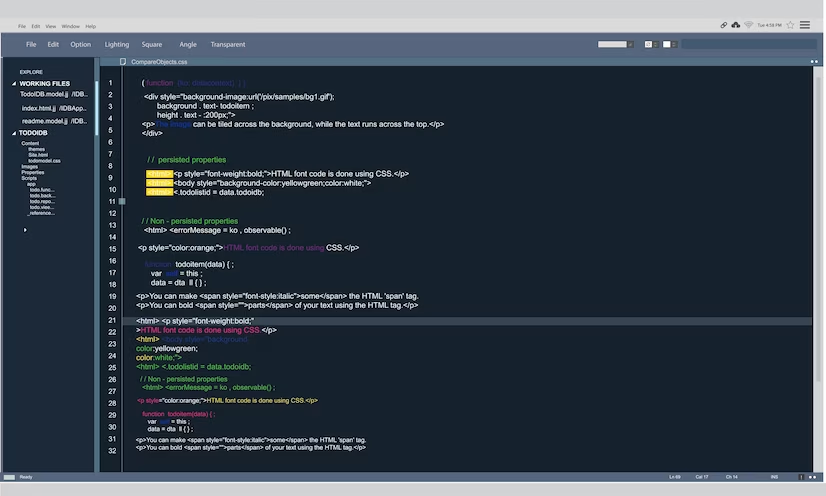
chr Function Python: Character Conversion and Manipulation
In the world of Python, character manipulation is a fundamental skill, especially when dealing with textual data. Python provides two powerful tools, the `chr()` and `ord()` functions, which play a pivotal role in working with characters and code points.
This comprehensive guide will lead you through mastering these functions, helping you decode characters, convert code points, and navigate the realm of text data effectively.
Understanding chr() and ord() Functions
The `chr()` and `ord()` functions are essential components of Python’s built-in functions. They facilitate the conversion between characters and Unicode code points, making it easier to work with textual data.
`chr()`: This function converts a Unicode code point into its corresponding character. It takes an integer (the code point) as input and returns the character represented by that code point.
– `ord()`: Conversely, `ord()` takes a character as input and returns its Unicode code point in the form of an integer.
Both functions are invaluable when dealing with character encoding, decoding, and text processing.
Getting Started with chr() and ord(): Basic Usage of chr() and ord()
Let’s explore the basic usage of these functions:
Using `chr()`
```python
# Using chr() to get the character 'A' (Unicode code point 65)
character = chr(65)
print(character) # Output: 'A'
```
Here, `chr(65)` returns ‘A’ since ‘A’ corresponds to Unicode code point 65.
Using `ord()`
```python
# Using ord() to get the Unicode code point of 'A'
code_point = ord('A')
print(code_point) # Output: 65
```
In this example, `ord(‘A’)` returns 65 since ‘A’ has a Unicode code point of 65.
Practical Tips for Using chr() and ord()
Converting Characters to Unicode Code Points
To convert characters back to their Unicode code points, you can use the `ord()` function:
```python
# Using ord() to get the Unicode code point of 'B'
code_point = ord('B')
print(code_point) # Output: 66
```
This is particularly useful for reverse operations, such as decoding.
Tip 2: Handling Special Characters
Both `chr()` and `ord()` are not limited to alphanumeric characters. They can handle a wide range of characters and symbols, including special characters. For instance:
```python
# Using chr() to get the copyright symbol '©' (Unicode code point 169)
copyright_symbol = chr(169)
print(copyright_symbol) # Output: '©'
```
If you are beginner in Python, check this tutorial and explore more about chr and ord
Let’s delve into more practical examples:
What is chr(65) in Python?
As we saw earlier, `chr(65)` returns ‘A’ because it corresponds to the Unicode code point 65.
What is chr(13) in Python?
The `chr(13)` function returns a carriage return character, often used to move the cursor to the beginning of a new line.
Handling Extended Unicode Characters
Both `chr()` and `ord()` are not limited to Basic Multilingual Plane (BMP) characters. They can handle characters beyond the BMP, which have code points greater than 65535. To represent such characters in Python strings, you can use escape sequences:
```python
# Representing characters with large code points using escape sequences
large_code_point_character = '\U0001F600' # Grinning Face Emoji
print(large_code_point_character) # Output: '😀'
```
In this example, the escape sequence `\U` followed by the Unicode code point in hexadecimal notation represents a character outside the BMP.
Use Cases for chr() and ord()
Understanding when and how to use `chr()` and `ord()` is essential. Here are some common use cases:
Character Encoding and Decoding
When working with text data, you’ll often need to encode and decode characters. These functions are invaluable for converting characters to code points and vice versa, ensuring proper communication between systems and applications.
Custom String Generation
You can use `chr()` to create custom strings with specific characters or symbols. For example, crafting a string with smiley emojis:
```python
# Creating a string with smiley emojis
smiley_string = chr(128512) + chr(128516)
print(smiley_string) # Output: '😀😄'
```
Working with Special Symbols
In scientific or mathematical applications, you may need to include special symbols or Greek letters. `chr()` allows you to insert these symbols into your documents or code effortlessly:
```python
# Using chr() to insert the Greek letter 'Σ' (Sigma) into a string
sigma_symbol = chr(931)
print(sigma_symbol) # Output: 'Σ'
```
Conclusion
Python `chr()` and `ord()` functions are indispensable tools for character manipulation, code point conversion, and text data processing. Whether you’re decoding characters, converting code points, generating custom strings, handling special symbols, or exploring characters beyond the BMP, these functions simplify these tasks.
With the knowledge gained from this guide, you’re well-prepared to leverage the power of `chr()` and `ord()` in your Python projects, enhancing your text-processing capabilities and confidently navigating the world of characters and symbols.
As you continue your Python journey, remember that character manipulation and encoding are crucial skills that open doors to various applications, from data analysis to natural language processing.
Average Rating