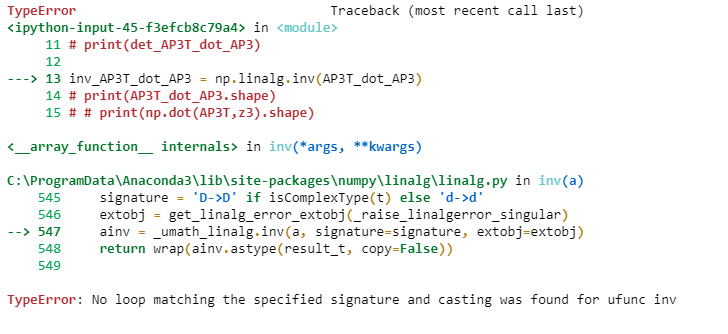
Matrix Inversion Python: Unlocking the Power
Matrix inversion is a fundamental operation in linear algebra with applications in various fields, including machine learning, physics, and engineering. In this comprehensive guide, we will explore how to calculate the inverse of a matrix using Python, focusing on both theory and practical implementation.
What is Matrix Inversion?
A matrix inverse is like a magic key that unlocks a range of mathematical possibilities. In simple terms, it allows us to reverse the effects of a matrix operation. To find the inverse of a matrix, we need to ensure it is square (having the same number of rows and columns) and non-singular (its determinant is not zero). Let’s dive into the methods and tools Python offers for this task.
Inverting a 2×2 Matrix in Python
In Python, you can calculate the inverse of a 2×2 matrix using a straightforward formula:
import numpy as np
matrix = np.array([[a, b],
[c, d]])
# Calculate the determinant
det = a*d - b*c
# Check if the matrix is invertible
if det != 0:
inverse_matrix = np.array([[d, -b],
[-c, a]]) / det
else:
print("Matrix is not invertible.")
This code calculates the determinant and checks if it’s non-zero before proceeding with inversion. This method works efficiently for 2×2 matrices.
Inverting a Matrix with NumPy in Python
For larger matrices, NumPy, a powerful library for numerical computations, simplifies the process. You can use the numpy.linalg.inv() function to find the inverse of a matrix:
import numpy as np
matrix = np.array([[a, b, c],
[d, e, f],
[g, h, i]])
inverse_matrix = np.linalg.inv(matrix)
How to Find the Inverse of a Matrix?
To summarize, finding the inverse of a matrix in Python involves:
- Ensuring the matrix is square and non-singular;
- Using the appropriate method based on the matrix’s size;
- Utilizing Python libraries like NumPy for efficient computation.
The Importance of Matrix Inversion
Matrix inversion plays a pivotal role in various scientific and engineering disciplines. Let’s explore some real-world applications:
Linear Systems and Equations
Inverting matrices is essential for solving linear systems of equations. For instance, consider a system of equations:
Ax = b
Here, ‘A’ is a coefficient matrix, ‘x’ is a vector of unknowns, and ‘b’ is a known vector. To find ‘x’, you can use matrix inversion:
x = A⁻¹ * b
Matrix inversion helps efficiently solve such systems, which arise in fields like physics, economics, and statistics.
Least Squares Problems
In machine learning and data analysis, least squares problems often involve finding the best-fitting line or curve. Matrix inversion aids in finding the parameters that minimize the sum of squared differences between observed and predicted values.
Eigenvalue Problems
Matrix inversion is closely related to finding eigenvalues and eigenvectors, which are crucial in various mathematical and scientific applications, including quantum mechanics, structural engineering, and image processing.
Computer Graphics and 3D Transformations
In computer graphics and 3D transformations, matrix inversion is employed to manipulate and render 3D objects accurately. This is vital for creating realistic visual effects in video games and simulations.
Optimization Problems
Optimization problems often require matrix inversion to determine critical points of extrema in a function. This is valuable in fields such as economics, engineering design, and logistics.
By understanding and applying matrix inversion techniques in Python, you open doors to solving complex problems in these and other domains.
Learn in this tutorial how to understanding matrix inversion.
Conclusion
In this comprehensive guide, we’ve explored the intricacies of matrix inversion in Python. From 2×2 matrices to 3D transformations, you’ve learned how to unlock the power of matrix operations. Python, with libraries like NumPy, provides a robust environment for performing these operations efficiently.
Matrix inversion is not just a mathematical concept; it’s a practical tool with applications spanning numerous disciplines. Whether you’re a data scientist, engineer, or mathematician, mastering matrix inversion in Python empowers you to tackle a wide array of real-world challenges.
So, go ahead and explore the endless possibilities of matrix mathematics in the Python ecosystem.
Average Rating