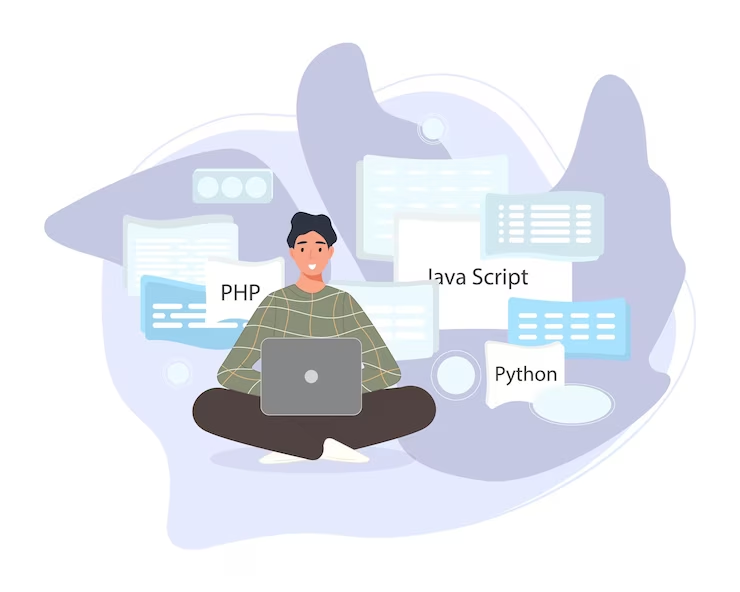
Python Cosine Similarity: Mastering for Practical Use
Welcome to our in-depth exploration of Python cosine similarity, a vital concept with broad applications in data analysis, text processing, and machine learning. In this comprehensive article, we aim to demystify cosine similarity, providing you with a deep understanding of its real-world uses and how to implement it using Python.
Whether you are new to programming or an experienced coder, this article equips you with the knowledge and skills needed to harness the potential of cosine similarity effectively.
Understanding Cosine Similarity
Core Concepts
Cosine similarity is a mathematical tool used to quantify the similarity between two non-zero vectors in a multi-dimensional space. Put simply, it helps us measure how similar or dissimilar two sets of data points are, making it invaluable in various fields.
The Essence of Cosine Similarity
Picture two vectors, A and B, in a multi-dimensional space. Cosine similarity between these vectors is represented by the cosine of the angle θ formed between them. The closer θ is to 0 degrees, the more similar the vectors are. If θ equals 90 degrees, indicating orthogonality, the vectors are dissimilar.
The Cosine Similarity Formula
Mathematically, cosine similarity can be expressed as follows:
cosine_similarity(A, B) = (A ⋅ B) / (∥A∥ * ∥B∥)
Where:
- A ⋅ B represents the dot product of vectors A and B;
- ∥A∥ and ∥B∥ denote the magnitudes (or norms) of vectors A and B, respectively.
Practical Applications of Cosine Similarity
Cosine similarity has a wide range of applications. Let’s delve into some key areas where it plays a significant role:
Text Similarity and Information Retrieval
In the realm of natural language processing (NLP), cosine similarity is extensively used to gauge the similarity between textual documents. This aids in tasks like document retrieval, plagiarism detection, and recommendation systems, making text comparisons efficient by representing documents as vectors.
Recommender Systems
Big players in e-commerce like Amazon and Netflix leverage cosine similarity to recommend products or movies to users. By analyzing user preferences and item descriptions as vectors, these platforms provide personalized recommendations, enhancing the user experience.
Learn more in the next tutorial:
Clustering and Classification
Cosine similarity is fundamental in clustering and classification tasks, helping group similar data points for pattern recognition and data organization.
Image Processing
In the field of computer vision, cosine similarity assists in image matching and retrieval. By converting images into feature vectors, visually similar images can be identified within large databases.
Implementing Cosine Similarity in Python
Now that we’ve covered the core concepts and practical applications of cosine similarity, let’s explore its Python implementation.
Calculating Cosine Similarity in Python
Python offers several libraries, including NumPy and Scikit-Learn, to compute cosine similarity efficiently. We’ll walk you through both methods, providing code examples for clarity.
Using NumPy
NumPy, a robust numerical computing library, simplifies cosine similarity calculations. Here’s a code snippet demonstrating its use:
import numpy as np
# Define two vectors, A and B
A = np.array([1, 2, 3])
B = np.array([4, 5, 6])
# Calculate cosine similarity
similarity = np.dot(A, B) / (np.linalg.norm(A) * np.linalg.norm(B))
Manual Implementation
For a deeper understanding of the underlying mathematics, you can implement cosine similarity manually:
def cosine_similarity(A, B):
dot_product = sum(a * b for a, b in zip(A, B))
magnitude_A = sum(a * a for a in A) ** 0.5
magnitude_B = sum(b * b for b in B) ** 0.5
return dot_product / (magnitude_A * magnitude_B)
Choosing the Appropriate Cosine Similarity Variant
TF-IDF (Term Frequency-Inverse Document Frequency)
In NLP, especially when working with text data, the TF-IDF representation is commonly used to calculate cosine similarity. TF-IDF considers term frequency and inverse document frequency to weigh the importance of terms in distinguishing documents. Scikit-Learn provides a straightforward TF-IDF vectorizer:
from sklearn.feature_extraction.text import TfidfVectorizer
# Sample documents
documents = ["This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"]
# Create TF-IDF vectors
tfidf_vectorizer = TfidfVectorizer()
tfidf_matrix = tfidf_vectorizer.fit_transform(documents)
# Calculate cosine similarity
cosine_sim = cosine_similarity(tfidf_matrix, tfidf_matrix)
Cosine Similarity for Sparse Data
When working with high-dimensional and sparse data, such as text data, it’s crucial to consider memory and computational efficiency. SciPy offers optimized functions for calculating cosine similarity on sparse matrices:
from scipy.spatial.distance import cosine
# Sparse matrices A and B
sparse_A = scipy.sparse.csr_matrix(A)
sparse_B = scipy.sparse.csr_matrix(B)
# Calculate cosine similarity
similarity = 1 - cosine(sparse_A, sparse_B)
Conclusion
We have embarked on an insightful journey into the world of Python cosine similarity. We started with the fundamentals, understanding how it quantifies the similarity between vectors in multi-dimensional spaces. We then explored its extensive applications, spanning text similarity, recommendation systems, clustering, and image analysis.
You’ve gained practical insights into implementing cosine similarity in Python, whether through the NumPy library or manual calculations. We also discussed specialized cases like TF-IDF and efficient handling of sparse data.
With this knowledge at your disposal, you are now well-prepared to tackle real-world data analysis, natural language processing, and machine learning tasks with confidence. Python cosine similarity is a versatile tool that can enhance the quality and efficiency of your projects. So, go ahead, experiment, and unlock its potential in your programming endeavors.
Average Rating