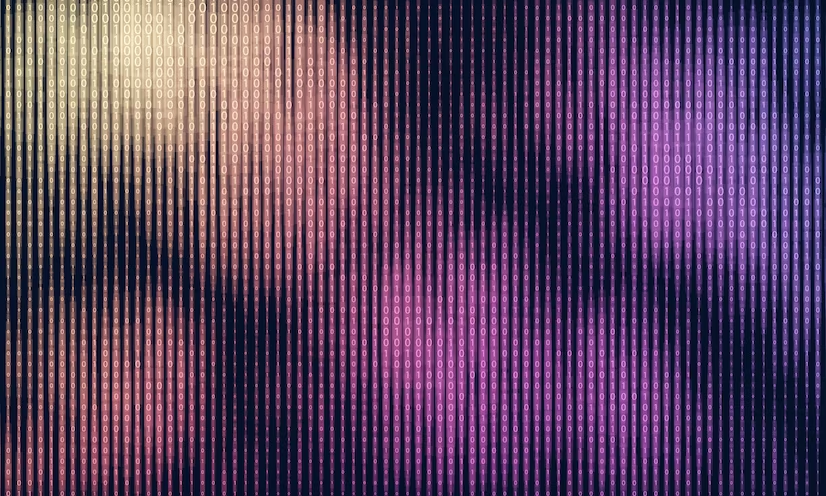
np.diag: Demystifying Diagonal Matrix Creation in Python
Diagonal matrices stand as fundamental elements in various mathematical and computer science disciplines, particularly in linear algebra and data analysis. While seemingly simple, these matrices wield immense power and find applications in diverse scenarios, from solving linear equations to conducting principal component analysis (PCA).
In this comprehensive guide, we embark on a journey to explore the creation, manipulation, and practical implementation of diagonal matrices in Python. Our focal point is the indispensable tool, np.diag, generously provided by the NumPy library.
What Are Diagonal Matrices?
A diagonal matrix is a square matrix where all elements outside the main diagonal are zero. This primary diagonal runs from the top-left to the bottom-right corner of the matrix. Typically denoted as “D,” it can be visually represented as follows:
D = | d1 0 0 |
| 0 d2 0 |
| 0 0 d3 |
Here, d1, d2, and d3 denote the diagonal elements, while the rest of the matrix remains populated with zeros. The beauty of diagonal matrices lies in their simplicity and their far-reaching applications across various mathematical operations.
Crafting Diagonal Matrices with np.diag
Leveraging np.diag
Python’s NumPy library furnishes us with np.diag, a potent function that streamlines the creation of diagonal matrices.
Here’s how you can wield its capabilities:
import numpy as np
# Creating a diagonal matrix from a list of diagonal elements
diagonal_elements = [2, 4, 6]
D = np.diag(diagonal_elements)
In this example, we initiate NumPy as np and craft a diagonal matrix D by harnessing np.diag. By feeding it a list of diagonal elements [2, 4, 6], we invoke the function’s prowess to construct the corresponding diagonal matrix.
Practical Prowess of Diagonal Matrices
Diagonal matrices serve as invaluable tools in a diverse array of mathematical and computational domains:
- Resolving Linear Systems
Diagonal matrices streamline the resolution of linear equations, elevating computational efficiency. In systems of equations cast as Ax = b, where A represents a diagonal matrix, the path to determining x becomes remarkably straightforward.
- Principal Component Analysis (PCA)
In the realms of data analysis and dimensionality reduction, PCA relies on diagonalization, wherein diagonal matrices take center stage. They facilitate the identification of a dataset’s principal components, effectively reducing its dimensionality while preserving essential information.
- Eigenvalue Decomposition
The realm of eigenvalue decomposition, a pivotal technique in linear algebra, often finds diagonal matrices at its core. This decomposition simplifies the analysis of matrix properties and transformations, rendering it a potent asset in various mathematical investigations.
- Spectral Graph Theory
In the captivating field of graph theory, diagonal matrices emerge as key players in spectral graph theory. They are instrumental in representing adjacency matrices and Laplacian matrices, affording their applications in diverse realms, including network analysis and image segmentation.
Operations on Diagonal Matrices
Diagonal matrices embrace a realm of straightforward operations:
- Addition and subtraction: Diagonal matrices of identical dimensions can be seamlessly added or subtracted element-wise;
- Scalar multiplication: The multiplication of a diagonal matrix by a scalar value cascades across all its diagonal elements, facilitating effective scaling;
- Transposition: The transpose of a diagonal matrix mirrors the original, preserving its structure;
- Inversion: A diagonal matrix proves invertible only when none of its diagonal elements are zero. Inverting such a matrix involves the reciprocation of each diagonal element.
The Art of Matrix Multiplication
Diagonal matrices wield a remarkable property when it comes to matrix multiplication. When a diagonal matrix intermingles with another matrix, the outcome manifests as a scaled version of the latter. This operation harbors tremendous potential in diverse mathematical transformations and simulations.
For instance, contemplate the union of a diagonal matrix D with another matrix A. The product DA orchestrates the scaling of each row within A by the corresponding diagonal element of D. Such an operation resonates profoundly in scenarios encompassing vector scaling, data transformation, or coordinate adjustments.
import numpy as np
# Birth of a diagonal matrix
D = np.diag([2, 3, 4])
# The arrival of another matrix
A = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# The entwining of matrices
result = D.dot(A)
The elements within each row of matrix A undergo rescaling based on the respective diagonal elements of D, ultimately yielding the transformed matrix “result.”
Diagonal matrices flaunt an efficiency prowess both in terms of storage and computation. Their predominance of zero elements translates into reduced memory footprint, a valuable trait when grappling with voluminous datasets or sparsely populated systems.
Moreover, many mathematical operations involving diagonal matrices unfurl with heightened efficiency, courtesy of their streamlined structure. Such efficiency injects substantial computational savings into a gamut of numerical algorithms.
Follow this video and discover more details
Conclusion
Diagonal matrices, the embodiment of simplicity and unique attributes, occupy a prominent niche within the realms of mathematics, computer science, and data analysis. Python, bolstered by the formidable np.diag function courtesy of NumPy, extends an accessible hand in the creation and manipulation of diagonal matrices, rendering them accessible for multifarious applications.
The mastery of diagonal matrices and their pivotal roles in linear systems, data analysis, and numerical computations bestows upon you a treasure trove of problem-solving tools and mathematical modeling capabilities.
Whether you’re embroiled in optimization challenges, machine learning endeavors, or simulations, the comprehension of diagonal matrices and their deft handling within the Python ecosystem will augment your skill set and usher in new vistas of possibility across your computational journey.
Average Rating