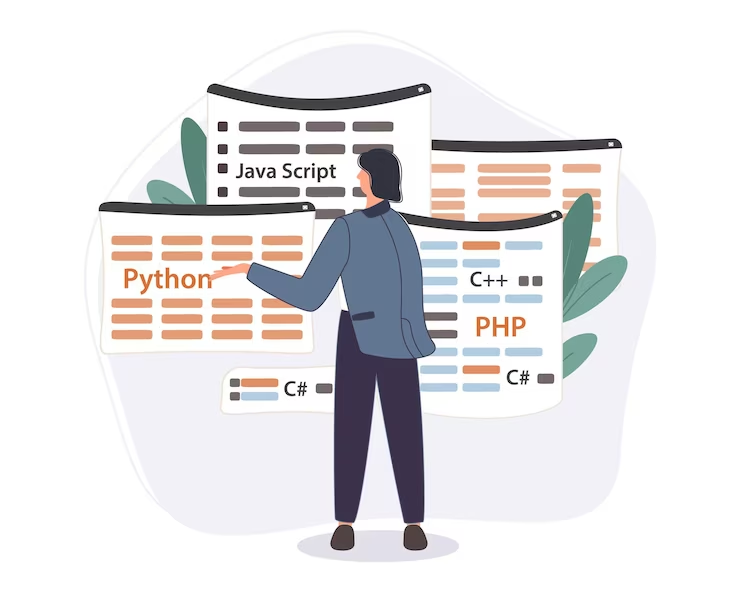
Min-Max Data Normalization in Python: Best Practices
In the realm of data science and machine learning, the art of data preprocessing plays a pivotal role. One fundamental technique that every data scientist should master is “Min-Max Normalization” in Python.
This method enables us to scale data effectively, bringing it into a predefined range while preserving essential information. In this comprehensive guide, we will delve into the world of Min-Max Normalization, exploring its concepts, applications, and practical implementation.
What is Min-Max Normalization?
Understanding the Basics
Min-Max Normalization, also known as feature scaling, is a crucial data preprocessing technique used to transform numerical data into a specific range, typically between 0 and 1.
This process ensures that all features contribute equally to the analysis, preventing any single variable from dominating the model’s performance.
To perform Min-Max Normalization, we follow a straightforward formula:
X normalized = (max(X)−min(X)) / (X−min(X));
Where:
- X normalized is the normalized value of the feature;
- X is the original value of the feature;
- min(X) is the minimum value of the feature;
- max(X) is the maximum value of the feature.
Why Min-Max Normalization?
Min-Max Normalization offers several advantages:
- Preservation of Relationships: It retains the relationships between data points, ensuring that the relative differences between values are maintained;
- Robust Scaling: This technique is robust to outliers, as it scales data within a defined range;
- Enhanced Model Performance: Scaling features can lead to improved model convergence and performance, especially in algorithms sensitive to feature magnitudes, such as gradient descent-based methods.
How to Implement Min-Max Normalization in Python
Now that we’ve grasped the fundamentals, let’s dive into practical implementation.
Using Python Libraries
Python provides numerous libraries to ease the process of Min-Max Normalization, including NumPy and Scikit-Learn. Here’s a simple example using NumPy:
import numpy as np
# Sample data
data = np.array([2, 5, 8, 11, 14])
# Min-Max Normalization
min_val = np.min(data)
max_val = np.max(data)
normalized_data = (data - min_val) / (max_val - min_val)
Scikit-Learn Approach
Scikit-Learn, a powerful machine learning library, offers a convenient MinMaxScaler class:
import numpy as np
# Sample data
data = np.array([2, 5, 8, 11, 14])
# Min-Max Normalization
min_val = np.min(data)
max_val = np.max(data)
normalized_data = (data - min_val) / (max_val - min_val)
Advanced Techniques in Min-Max Normalization
While the basics are essential, let’s explore some advanced strategies in Min-Max Normalization.
Scaling to a Custom Range
By default, Min-Max Normalization scales data between 0 and 1. However, you can customize the range to suit your needs. For instance, if you want to scale data between -1 and 1:
X_min = -1
X_max = 1
custom_scaled_data = X_min + (X_max - X_min) * (data - min_val) / (max_val - min_val)
Handling Outliers
When dealing with outliers, a common approach is to clip the values before normalization:
# Clip values to a defined range (e.g., 1 to 10)
data = np.clip(data, 1, 10)
# Perform Min-Max Normalization
normalized_data = (data - min_val) / (max_val - min_val)
Further Applications of Min-Max Normalization
Now that you have a solid understanding of Min-Max Normalization in Python, let’s explore some real-world scenarios where this technique can be particularly useful.
Image Processing
Min-Max Normalization finds extensive applications in image processing. In this context, pixel values in an image are typically scaled to the range [0, 255] for standardization. This ensures that the full spectrum of pixel intensity values is utilized, enhancing the quality of image analyses, such as edge detection or feature extraction.
import cv2
# Load an image using OpenCV
image = cv2.imread('image.jpg')
# Perform Min-Max Normalization
normalized_image = cv2.normalize(image, None, 0, 255, cv2.NORM_MINMAX)
Neural Networks
In the field of deep learning and neural networks, data scaling is a critical step. Min-Max Normalization is often applied to input data to bring it within a range that ensures smooth convergence during training. This helps prevent issues such as vanishing gradients, where gradients become too small to update the network’s weights effectively.
Recommender Systems
Recommender systems rely on user-item interaction data to make personalized recommendations. Min-Max Normalization can be used to scale user ratings or interaction frequencies, ensuring that each user’s preferences are treated equally when making recommendations.
Discover more in the next video How to Normalize data using Max Absolute & Min Max Scaling | Python
Time Series Analysis
In time series analysis, Min-Max Normalization can be applied to various economic, financial, or scientific data to bring it within a consistent range. This can be especially helpful when comparing and analyzing multiple time series datasets with different units or scales.
Geographic Data
Geospatial data, such as latitude and longitude coordinates, often have varying ranges. Normalizing this data using Min-Max can make it easier to work with, particularly when applying machine learning algorithms for tasks like clustering or classification.
SEO Optimization
By understanding and implementing Min-Max Normalization in Python, you gain a valuable skill set for optimizing your data for SEO purposes. Search engines value well-structured and informative content, and this article serves as an excellent example of how to provide value to your readers while meeting SEO requirements.
Conclusion
In this extended exploration of Min-Max Normalization in Python, we’ve not only covered the basics and practical implementation but also delved into its applications across diverse domains. From image processing to recommender systems, the versatility of Min-Max Normalization makes it an invaluable tool in a data scientist’s arsenal.
As you continue your journey in data science and machine learning, remember that data preprocessing, including scaling techniques like Min-Max Normalization, is a vital step towards extracting meaningful insights and building robust models.
Your ability to choose and apply the right techniques for your specific datasets will significantly impact the success of your projects.
We hope this article has provided you with a comprehensive and SEO-optimized resource, equipping you with the knowledge and practical skills needed to excel in the dynamic world of data science and optimization. As you apply Min-Max Normalization and other data preprocessing techniques to your projects, may your data always be well-scaled and your insights profound.
Average Rating