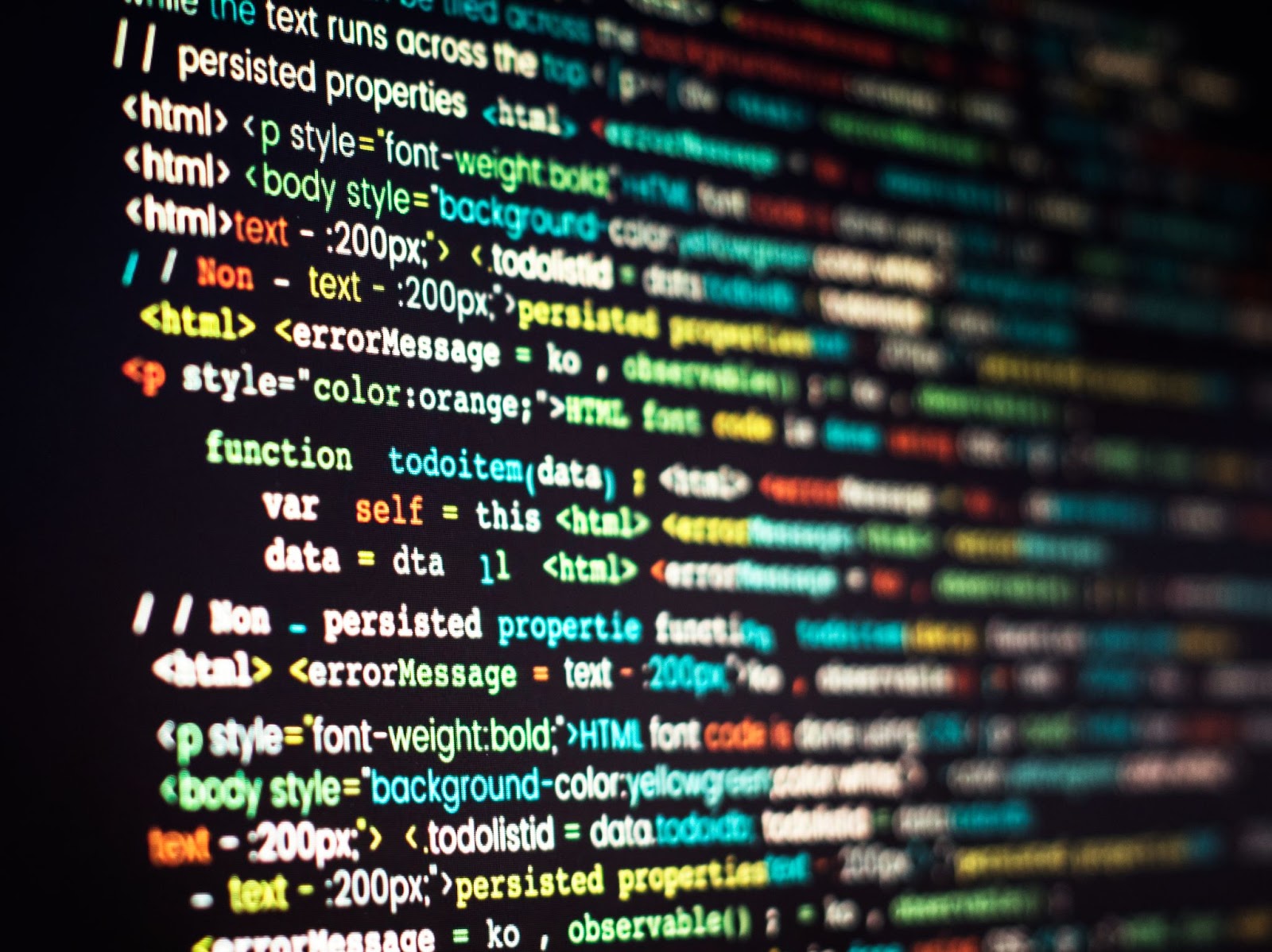
Exploring Python’s Lambda Functions for Multiple Parameters
Python, renowned for its simplicity and versatility, offers programmers a plethora of tools to streamline code and enhance productivity. Among these tools, lambda functions stand out as a concise yet powerful feature. Also known as anonymous functions, provide a means to create small, throwaway functions without the need for a formal definition. This article will be your guide into the world of it, delving into their syntax, use cases, and practical examples. Whether you’re a seasoned developer or just beginning your coding journey, this exploration will equip you with the knowledge and skills to leverage it effectively in your projects. So, let’s dive in and uncover the hidden potential of this essential feature.
It represents distinctive constructs within the Python programming language. In contrast to conventional functions established using the ‘def’ keyword, they exhibit brevity and are tailored for uncomplicated operations. As their very nature suggests, they have the capability to accept diverse arguments. Nevertheless, they are confined to the execution of a single expression.
The general structure of a lambda function is as follows:
lambda arguments: expression
Using a Single Argument
These functions possess remarkable versatility; even when supplied with just one argument, their potential for streamlining code remains formidable. Imagine a situation where it must augment any given number by 5. Rather than crafting an extensive, multi-line function, this same outcome can be effortlessly accomplished through the utilization of a lambda.
Here’s how to craft it for this task:
# Defining a lambda function to add 5 to any provided number
add_five = lambda x: x + 5
# Testing the function with the value 3
result = add_five(3)
print(result) # This will output: 8
When executed, the result will display the value 8, as the lambda function added 5 to the initial value of 3.
Using Multiple Arguments
Lambda functions possess the capability to extend beyond single arguments, showcasing their prowess in handling multiple inputs and yielding efficient outcomes. When confronted with computational tasks demanding the manipulation of more than one input value, such as the multiplication of two numbers, lambda functions prove to be invaluable tools.
Here’s a demonstration using a lambda function to multiply two numbers:
# Crafting a lambda function to multiply two provided numbers
multiply = lambda x, y: x * y
Let’s explore the practicality of it in Python through an illustrative example. Suppose we wish to evaluate the product of two numbers, 3 and 5. We can achieve this elegantly with the following code snippet:
result = lambda x, y: x * y
print(result(3, 5)) # Output: 15
Upon executing this code, the result will be 15. The lambda function, concise in its nature, swiftly multiplies the values 3 and 5, providing an efficient solution for this specific task.
In the realm of Python programming, it is often referred to as “anonymous functions,” finding their niche. They manifest as lightweight, transient constructs that facilitate rapid definition without the need for a cumbersome function declaration. Their true potential becomes evident when they synergize with other Python functions, with map() and sorted() being noteworthy collaborators, elevating the capabilities of these functions to new heights.
Let’s delve into how lambda functions seamlessly integrate with the map() function:
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, numbers))
In this instance, we employ map() alongside a lambda function to square each element within the ‘numbers’ list. The result, stored in the ‘squared’ variable, will be a list containing the squared values of the original elements. This succinct combination of lambda and map illustrates their synergy, enhancing code conciseness and clarity.
The versatility of lambda functions extends beyond single arguments, as they can be adapted to handle multiple parameters. By grasping the nuances of lambda functions, you unlock the potential to streamline your code and enhance its readability, making your Python programming endeavors more efficient and effective
In a bird’s-eye view:
Python’s map() function offers a conduit to apply a specific function to each element within an iterable, such as a list. When amalgamated with a lambda function, this union paves the way for expeditious and resource-efficient transformations.
Example:
Assume there’s a requirement to add 5 to every number in a given list. Instead of looping through the list and performing the operation, map() and a lambda function can be used for a more concise solution.
# Initialize a list of numbers.
nums = [1, 2, 3]
# Employ the lambda function via map to increase each number by 5.
mapped_numbers = map(lambda x: x + 5, nums)
# Since the map function returns a map object, convert it back to a list for better readability.
final_result = list(mapped_numbers)
# Display the modified list.
print(final_result) # Expected output: [6, 7, 8]
Employing Lambda Functions with sorted()
Python’s sorted() function enables the sorting of iterables. While it can easily sort basic data structures like lists, for complex data structures such as lists of tuples, a custom sorting mechanism may be required. This is where lambda functions shine by serving as the ‘key’ argument to influence the sorting criteria.
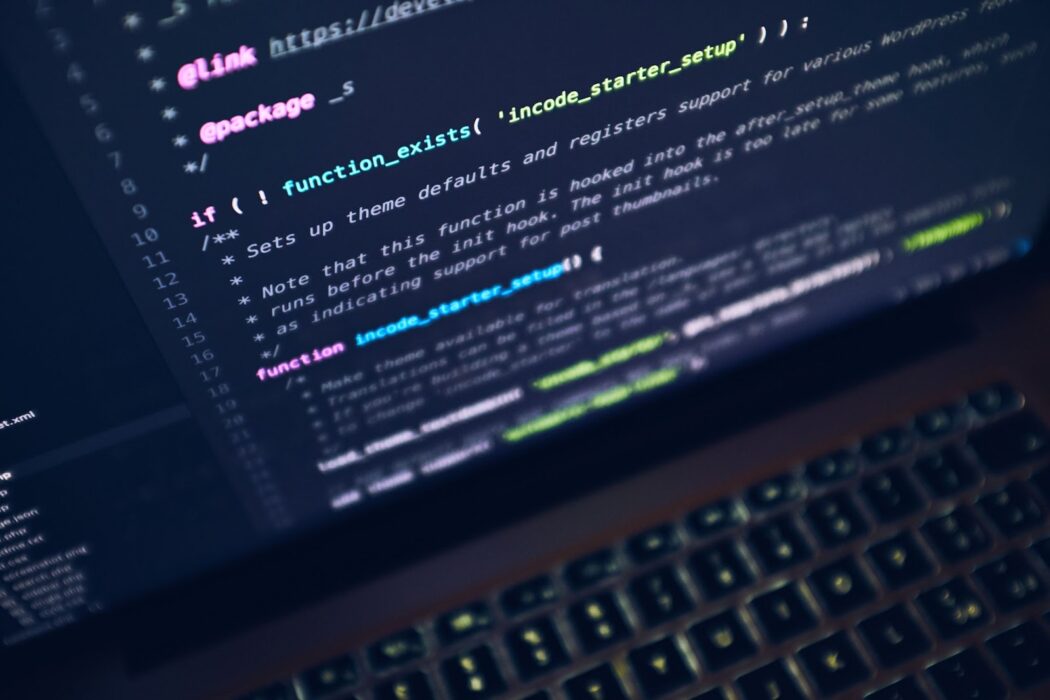
Example:
Imagine having a list of tuples representing fruits and their respective prices. The goal is to organize this list by fruit prices in ascending order.
# Create a list of tuples showcasing fruits and their respective costs.
fruit_prices = [('Banana', 3), ('Apple', 1), ('Orange', 5)]
# Use the sorted function, and provide a lambda function to sort the tuples by the price (second element of each tuple).
organized_by_price = sorted(fruit_prices, key=lambda fruit: fruit[1])
# Exhibit the organized list.
print(organized_by_price) # Expected output: [('Apple', 1), ('Banana', 3), ('Orange', 5)]
With the lambda function in place, the list is systematically sorted based on price, facilitating easy comprehension and subsequent processing.
In essence, the adaptability of lambda functions, especially when amalgamated with functions like map() and sorted(), enhances the efficiency of Python code by making operations more succinct and readable.
Conclusion
In this piece, we delved into the realm of Python lambda functions, elucidating their practical applications.
With a grasp of their fundamental utility, you can now embark on a journey to hone your skills, applying it to various iterable data structures to tackle multifaceted scenarios.
Should you find yourself inquisitive or brimming with insights, we warmly welcome your comments below. Share your questions or proffer suggestions for refining our discourse; your input is invaluable.
Average Rating