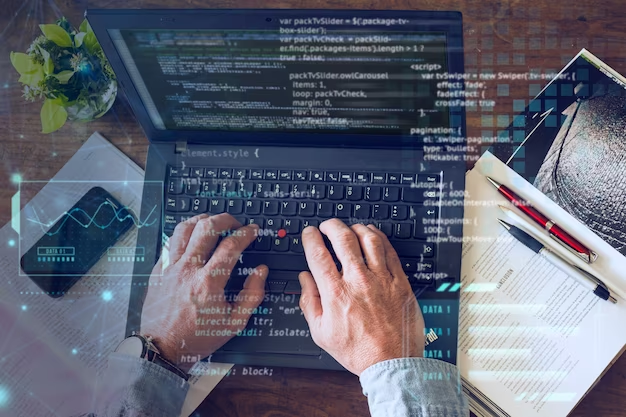
Python Age Calculator: Guide For You
In this tutorial, we’ll dive into creating a simple Python program to calculate a user’s age. Whether you’re a beginner just starting to learn Python or someone looking to embark on their first small project, this tutorial is designed for you. We’ll harness the power of Python’s built-in datetime module to accomplish this task, so there’s no need to install any additional dependencies.
Building the Age Calculator in Python
Let’s begin by importing the necessary module:
# Import the required dependencyfrom datetime import date |
Next, we’ll prompt the user to input their birthday and create a datetime object with that information:
# Ask the user to input year, month, and day of their birthday# and output a datetime object of their birthdaydef get_user_birthday(): birth_year = int(input(‘Please enter your birth year: ‘)) birth_month = int(input(‘Please enter your birth month: ‘)) birth_day = int(input(‘Please enter your birth day: ‘)) # Convert user input into a datetime object user_birthday = date(birth_year, birth_month, birth_day) return user_birthday |
Now, we can proceed to calculate the user’s age:
# Define a function to calculate the user’s agedef calculate_age(user_birthday): # Get the current date today = date.today() # Calculate the years difference year_diff = today.year – user_birthday.year # Check if the birth month and birth day precede the current month and current day precedes_flag = ((today.month, today.day) < (user_birthday.month, user_birthday.day)) # Calculate the user’s age age = year_diff – precedes_flag # Return the age value return age |
Finally, we can execute the code:
if __name__ == “__main__”: user_birthday = get_user_birthday() current_age = calculate_age(user_birthday) print(f”Your age is: {current_age}”) |
Testing the code with a sample birthday:
Please enter your birth year: 1999Please enter your birth month: 02Please enter your birth day: 01 |
You should get:
Your age is: 23 |
Complete Code
Here’s the complete code for your reference:
# Import the required dependencyfrom datetime import date # Ask the user to input year, month, and day of their birthday# and output a datetime object of their birthdaydef get_user_birthday(): birth_year = int(input(‘Please enter your birth year: ‘)) birth_month = int(input(‘Please enter your birth month: ‘)) birth_day = int(input(‘Please enter your birth day: ‘)) # Convert user input into a datetime object user_birthday = date(birth_year, birth_month, birth_day) return user_birthday # Define a function to calculate the user’s agedef calculate_age(user_birthday): # Get the current date today = date.today() # Calculate the years difference year_diff = today.year – user_birthday.year # Check if the birth month and birth day precede the current month and current day precedes_flag = ((today.month, today.day) < (user_birthday.month, user_birthday.day)) # Calculate the user’s age age = year_diff – precedes_flag # Return the age value return age if __name__ == “__main__”: user_birthday = get_user_birthday() current_age = calculate_age(user_birthday) print(f”Your age is: {current_age}”) |
More Precise Method
The more precise method calculates the age considering the birth year, month, and day, making it more accurate.
Now, let’s implement both methods and see the differences.
Method | Calculation | Precision |
---|---|---|
Simple Method | Current Year – Birth Year | Less Precise |
Precise Method | Current Date – Birth Date | More Precise |
Video Explanation
In order to explain this topic in more detail, we have prepared a special video for you. Enjoy watching it!
Conclusion
In this tutorial, we explored two methods for calculating your age using Python. You can choose between a simple method for a quick result or a more precise method for accuracy. Feel free to leave comments below if you have any questions or suggestions for improvements. Happy coding!
Average Rating