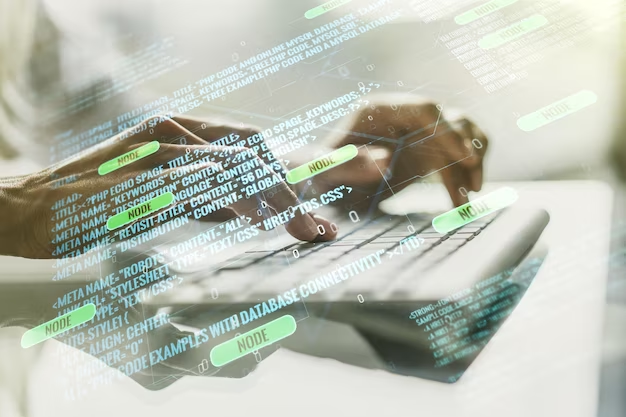
PyShark: What It Is And How To Use
 In the world of network programming and analysis, efficient packet parsing is essential. Python offers various libraries for this purpose, and PyShark stands out as a unique option. This article explores PyShark, a Python wrapper for tshark, Wireshark’s command-line utility. PyShark enables Python developers to parse packets using Wireshark dissectors, providing a powerful tool for network analysis.
What Is PyShark?
PyShark is a Python utility and library designed to parse packets using Wireshark dissectors. Unlike some other packet parsing modules, PyShark doesn’t directly parse packets; instead, it leverages tshark’s ability to export XMLs and uses them for parsing. This approach allows PyShark to use all installed Wireshark dissectors, making it a versatile choice for network analysis.
Installation
Before diving into PyShark, you need to install it. PyShark supports Python 3.7 and higher. You can install it using pip with the following command:
pip install pyshark |
Alternatively, you can clone the PyShark repository from GitHub and install it manually:
git clone https://github.com/KimiNewt/pyshark.gitcd pyshark/srcpython setup.py install |
Usage
Reading from a Capture File
One common use case for PyShark is parsing packets from a capture file. Here’s how you can do it:
import pyshark cap = pyshark.FileCapture(‘/path/to/your/capture_file.cap’)for packet in cap: print(packet) |
Reading from a Live Interface
PyShark can also capture packets from a live network interface. Here’s an example:
capture = pyshark.LiveCapture(interface=’eth0′)capture.sniff(timeout=50) for packet in capture: print(‘Just arrived:’, packet) |
Filtering Packets
PyShark allows you to filter packets, either when reading from a capture file or a live interface. You can use BPF (Berkeley Packet Filter) filters or display filters to narrow down the packets you’re interested in. Here’s an example using a display filter:
filtered_cap = pyshark.FileCapture(‘/path/to/your/capture_file.cap’, display_filter=’http’)for packet in filtered_cap: print(packet) |
Accessing Packet Data
Accessing packet data is straightforward with PyShark. Packets are divided into layers, and you can access their attributes. For instance:
packet[‘ip’].dst # By protocol stringpacket.ip.src # By protocol attributepacket[2].src # By layer index |
You can also use the dir(packet.my_layer) command to see available attributes for a layer.
Decrypting Packet Captures
PyShark supports automatic decryption of traces using standards like WEP, WPA-PWD, and WPA-PSK. For example:
capture = pyshark.FileCapture(‘/path/to/your/encrypted_capture.cap’, decryption_key=’your_decryption_key’)for packet in capture: print(packet) |
Comparison Table
Feature | PyShark | Scapy | dpkt |
---|---|---|---|
Parsing from Capture | Supported | Supported | Supported |
Parsing from Live | Supported | Supported | Supported |
Installation | Easy (pip install pyshark) | Easy (pip install scapy) | Easy (pip install dpkt) |
Protocol Support | Wireshark Dissectors | Custom Parsing and Crafting | Custom Parsing |
Filtering Capabilities | Display Filters and BPF Filters | Custom Filters and Conditions | Custom Filters and Conditions |
Decryption Support | WEP, WPA-PWD, WPA-PSK | Not Built-in | Not Built-in |
Layer-Based Parsing | Yes | Yes | Yes |
Active Development | Yes | Yes | Limited |
Compatibility | Cross-platform | Cross-platform | Cross-platform |
Here’s a comparative table outlining key features of PyShark, Scapy, and dpkt for packet parsing and analysis in Python. Each library has its strengths and use cases, so choosing the right one depends on your specific needs and preferences.
Video Explanation
In order to explain this topic in more detail we have prepared a special video for you. Enjoy watching it!
Key Advantages of PyShark
- User-Friendly: PyShark offers a straightforward and user-friendly interface for parsing and analyzing network packets, making it suitable for both beginners and experienced developers;
- Wireshark Integration: PyShark leverages the power of Wireshark dissectors, allowing you to access detailed information about various network protocols effortlessly;
- Cross-Platform: It works seamlessly on both Windows and Linux operating systems, providing flexibility in your choice of development environment;
- Decryption Support: PyShark supports automatic decryption of traces using encryption standards such as WEP, WPA-PWD, and WPA-PSK;
- Active Development: The library is actively maintained, ensuring that it stays up-to-date with the latest developments in network protocols and technologies;
- Layer-Based Parsing: You can access packet data at different layers, simplifying the process of extracting information from complex network traffic;
- Filtering Capabilities: PyShark supports both display filters and BPF filters, enabling you to focus on specific packet subsets for in-depth analysis;
- Versatile Usage: Whether you’re reading from a capture file, a live interface, or a remote interface, PyShark provides the necessary tools to handle various scenarios;
- Protocol Support: It covers a wide range of protocols thanks to Wireshark’s extensive dissectors, making it suitable for diverse network analysis tasks;
- Ease of Installation: Installing PyShark is straightforward, as it can be easily installed using the pip package manager;
- Community and Documentation: PyShark benefits from an active community of users and has extensive documentation available to assist users in getting started and troubleshooting.
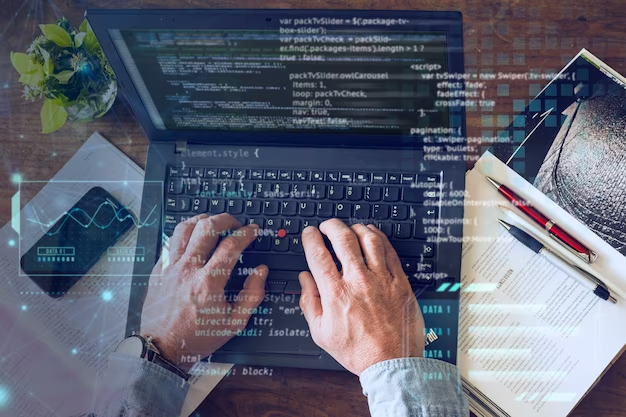
Conclusion
In conclusion, PyShark stands as a versatile and powerful Python library for network packet parsing and analysis. Its integration with Wireshark’s dissectors grants users access to detailed network protocol information, simplifying the often complex task of network analysis. With cross-platform compatibility, PyShark can be seamlessly employed on both Windows and Linux systems, offering flexibility to developers.
One of its standout features is its support for automatic decryption of traces using encryption standards like WEP, WPA-PWD, and WPA-PSK. This capability enhances its utility for various network security and monitoring applications.
Moreover, PyShark provides a user-friendly interface that accommodates both novice and experienced users. Its layer-based parsing approach allows for precise data extraction from network traffic, and the filtering capabilities, including display and BPF filters, enable focused analysis.
FAQ
PyShark is a Python wrapper for TShark, leveraging Wireshark’s powerful dissectors to parse network packets. Unlike other packet parsing libraries, PyShark doesn’t parse packets itself; instead, it utilizes TShark’s ability to export XML data for parsing. This approach allows PyShark to provide extensive protocol support without needing to reinvent the wheel.
PyShark supports Python 3.7 and above. There is also a legacy version called “pyshark-legacy” available for Python 2.
Yes, PyShark can capture live network traffic from a specified interface. It does this by invoking TShark with the selected interface to capture packets in real time. Users can apply display filters to focus on specific traffic.
PyShark offers automatic decryption support for network traces using encryption standards such as WEP, WPA-PWD, and WPA-PSK. By specifying the encryption type and key, PyShark can decrypt captured encrypted traffic for analysis.
Average Rating