Building a Currency Converter Program in Python
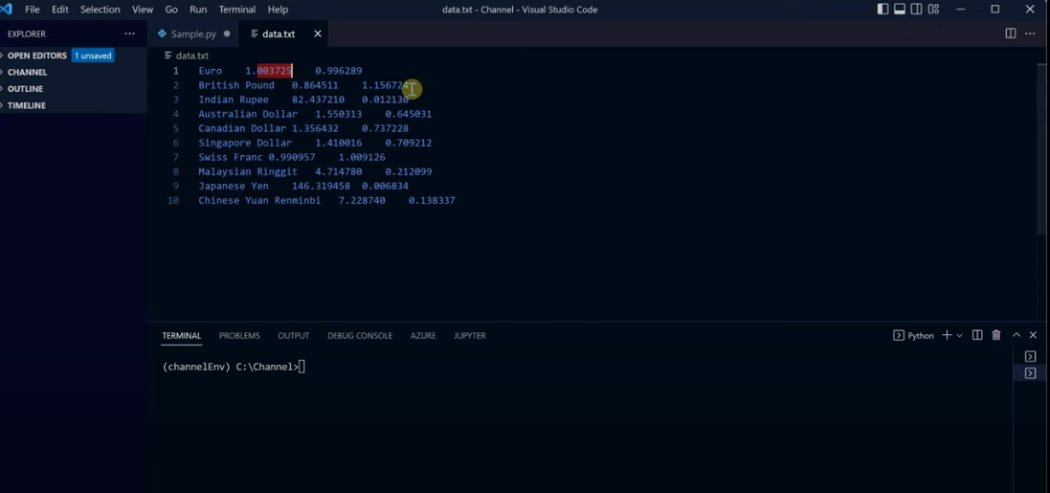
<alt=”creating a currency converter in Python ”/>
In this guide, we will walk you through creating a currency conversion program in Python using the ExchangeRate-API. This is an ideal project for beginners in Python.
<iframe width=”560″ height=”315″ src=”https://www.youtube.com/embed/snPGUT-Fxa4?si=gFSi59AufOA6K5NH” title=”YouTube video player” frameborder=”0″ allow=”accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture; web-share” allowfullscreen></iframe>
How do you convert currencies in Python?
To get started, make sure you have the ‘requests’ library installed:
pip install requests
Creating an API Key
We will be working with ExchangeRate-API, a convenient and reliable API for handling currency exchange rates. To obtain a free API key, follow these steps:
- Go to the website’s homepage and enter your email;
- Click the “Get Free Key!” button and set a password;
- After approximately 30 seconds, check your email for a confirmation link;
- Click the link to access the control panel and view your API key;
- Now, create a file named ‘credentials.py’ in your project directory and save your API key in it.
Building the Currency Converter Program
- Let’s begin by importing the necessary modules:
import requests from credentials import api_key
- Next, we’ll define a function to gather user input for currency conversion:
def get_user_input(): curr_from = input('Please enter the currency from which you would like to convert: ').upper() curr_to = input('Please enter the currency to which you would like to convert: ').upper() amount = int(input('Please enter the amount you would like to convert: ')) return curr_from, curr_to, amount
- Now, let’s define the URL for the GET request to perform currency conversion:
def get_converted_amount(curr_from, curr_to, amount): url = f'https://v6.exchangerate-api.com/v6/{api_key}/pair/{curr_from}/{curr_to}/{amount}' data = requests.get(url).json() converted_amount = data['conversion_result'] return converted_amount
- Finally, execute the program:
if __name__ == '__main__': curr_from, curr_to, amount = get_user_input() converted_amount = get_converted_amount(curr_from, curr_to, amount) print(f'{amount} {curr_from} = {converted_amount} {curr_to}')
What Is the Objective of a Currency Converter in Python?
The objective of creating a currency converter program in Python is to build a tool capable of performing the following tasks:
- Currency Conversion: The primary goal is to convert an amount from one currency to another based on the current exchange rate. This allows users to quickly determine the equivalent value of their money, aiding in financial planning and international transactions;
- User-Friendly Interface: The converter should have an intuitive and user-friendly interface, prompting users to input the source currency, target currency, and the amount they want to convert. This ensures ease of use and accessibility for a wide range of users;
- Real-Time Exchange Rates: To ensure accuracy in conversion, the converter should have access to real-time currency rate data. This is often achieved through integration with currency rate APIs, as demonstrated in the example with ExchangeRate-API;
- Error Handling: The program should gracefully handle potential errors, such as invalid currency codes or network issues when fetching currency rate data. In case of such errors, the program should provide clear feedback to the user;
- Modularity and Reusability: Developing the converter as modular code with the potential for reuse allows for integration into other applications or adaptation for various currency conversion tasks;
- Educational Tool: For beginners learning Python, creating a currency converter serves as a practical project to apply programming concepts. It helps learners understand topics such as user input, data parsing, API integration, and basic arithmetic operations.
Thus, the goal of creating a currency converter in Python is to develop a versatile and convenient tool that facilitates currency conversion while simultaneously promoting the understanding and application of programming concepts. It combines practical utility with educational value.
What Is the Currency Library in Python?
As of my last knowledge update in September 2021, Python does not have a built-in standard library specifically designed for working with currencies or currency conversion. Operations related to currency are typically implemented using external libraries or custom code.
To address such tasks in Python, developers often rely on third-party libraries or APIs that provide currency rate data and currency conversion functionalities. These libraries can be integrated into Python projects to streamline various currency-related operations.
Among the popular third-party libraries and APIs used for currency handling and conversion in Python are:
- Forex-python: This is a widely used Python library for working with currency exchange rates and performing conversions. It allows fetching currency rate data from various sources and executing currency conversions. GitHub Repository: https://github.com/MicroPyramid/forex-python;
- Open Exchange Rates: Open Exchange Rates is a well-known API that offers real-time currency rate data. You can access this API using Python to obtain currency rate data and perform conversions. API Documentation: https://docs.openexchangerates.org/docs/python;
- ExchangeRate-API: ExchangeRate-API, as mentioned earlier, provides an API for currency rate data. With it, you can fetch currency rates and perform conversions in Python. API Documentation: https://www.exchangerate-api.com/docs/python-currency-api.
Please note that the availability and popularity of libraries and APIs can change over time. When working on currency-related projects in Python, it’s advisable to check for the latest versions and documentation.
Conclusion
In this tutorial, we embarked on an exciting journey to create a powerful currency converter program in Python. We achieved several key objectives:
- Conversion Convenience: We built a user-friendly interface that allows users to easily convert currencies, making financial transactions across countries more straightforward;
- Real-Time Exchange Rates: Through integration with ExchangeRate-API, we ensured accurate and up-to-date currency rate information, enabling precise conversions;
- Educational Experience: This project is not only practical but also educational, making it an excellent choice for Python beginners. We delved into fundamental programming concepts while simultaneously creating a useful tool;
- Modularity and Reusability: The code we developed can be easily incorporated into other projects or adapted for various currency conversion tasks, enhancing its modularity and reusability.
As you continue your journey in Python programming, you can explore more complex projects and expand your programming skills. Feel free to explore our other Python programming articles, and if you have questions or suggestions for improvement, don’t hesitate to share your thoughts. Happy coding!
Average Rating