Binomial Distribution in Python: Explained and Implemented
To proceed with this tutorial, one needs to have specific Python libraries installed: scipy, numpy, and matplotlib. If these are not already installed, you can install them using the following commands in the Command Prompt on Windows:
```bash
pip install scipy
pip install numpy
pip install matplotlib
```
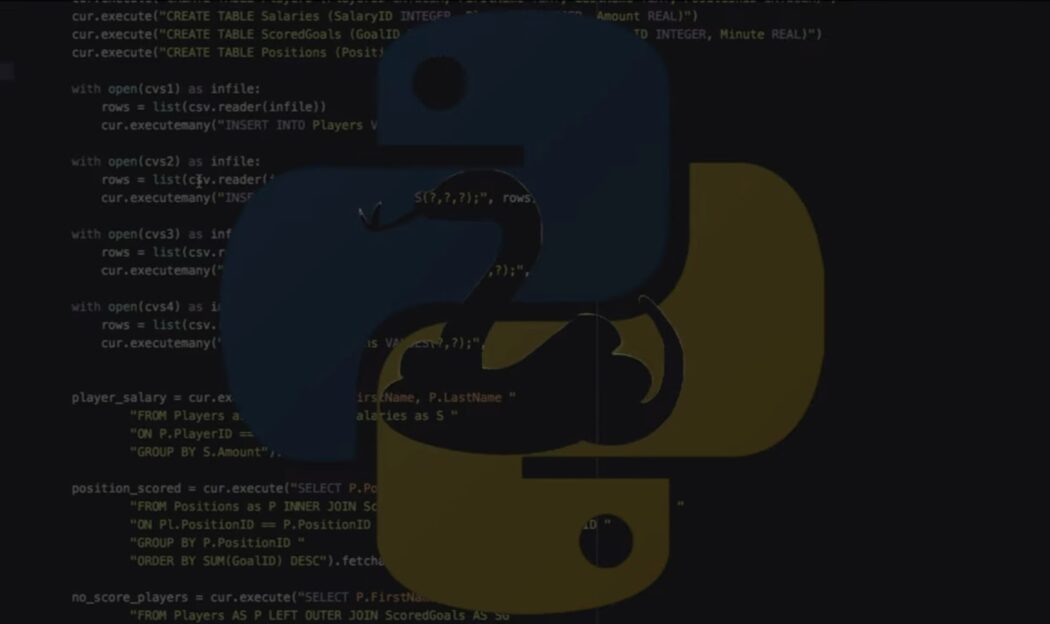
Understanding Success and Failure Probabilities
If the probability of success is denoted as p, then the probability of failure is given by q=1−p. Consequently, the probability of achieving k successes and (n−k) failures can be calculated as:
\[p^k \times (1−p)^{n−k}\]
The number of ways to achieve k successes is determined by the combination formula:
\[\frac{n!}{(n−k)! \times k!}\]
Using these notations, we can derive a probability mass function (PMF) for the total probability of achieving k successes in n experiments:
\[f(k;n,p)=Pr(k;n,p)=Pr(X=k)=\frac{n!}{(n−k)! \times k!} \times p^k \times (1−p)^{n−k}\]
A probability mass function (PMF) is a function that indicates the probability that a discrete random variable will have a particular value.
Additionally, the formula for the binomial cumulative probability function is:
\[F(k;n,p)=\sum_{i=0}^{x}\frac{n!}{(n−i)! \times i!} \times p^i \times (1−p)^{n−i}\]
Binomial Distribution Example
Imagine you are rolling a standard 6-sided die 12 times, aiming to calculate the probability of obtaining the number 3 as an outcome 5 times. In this scenario, rolling a 3 constitutes a success, while rolling any other number (1, 2, 4, 5, 6) is considered a failure. On each roll, the probability of getting a 3 is \(\frac{1}{6}\).
- Based on these assumptions, you would expect to obtain a 3 as an outcome 2 times out of the 12 rolls (\(12 \times \frac{1}{6}\)). But how can you determine the probability of observing 3 as an outcome 5 times?;
- Using the previously mentioned formula, you can calculate it precisely. Given that the experiment is repeated 12 times (n = 12), the desired number of outcomes is 5 (k = 5), and the probability is approximately 0.17 (p = 0.17), you can substitute these values into the equation;
- \[Pr(5;12,0.17)=Pr(X=5)=\frac{12!}{(12−5)! \times 5!} \times 0.17^5 \times (1−0.17)^{12−5} \approx 0.03\]
This probability represents the p-value for a significance test. Since 0.03 is less than the typical significance level of 0.05, you would reject the null hypothesis and conclude that the die is biased toward showing the number 3.
Creating and Visualizing Binomial Distribution in Python
Now, let’s delve into how to generate binomial distribution values and visualize them using Python, utilizing the numpy, matplotlib, and scipy libraries.
First, import the necessary modules:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import binom
```
Next, define your experiment parameters based on the previous example:
```python
n = 12
p = 0.17
x = np.arange(0, n+1)
```
Here, x is an array representing the number of times any number can be observed. With this data, you can calculate the binomial probability mass function (PMF), which describes the probability of observing each value in the distribution:
```python
binomial_pmf = binom.pmf(x, n, p)
print(binomial_pmf)
```
You will obtain an array with 13 values, corresponding to the probabilities for each x value. Finally, you can visualize the binomial distribution using matplotlib:
```python
plt.plot(x, binomial_pmf, color='blue')
plt.title(f"Binomial Distribution (n={n}, p={p})")
plt.show()
```
The resulting graph will display the probabilities associated with each possible outcome.
Understanding the Visualization
Interpreting the graph, you can observe that if you select any number from 1 to 6 (representing the sides of the die) and roll the die 12 times, the highest probability is for that number to appear 2 times.
In simpler terms, if you choose, for instance, the number 1 and roll the die 12 times, you are most likely to see it appear twice. If you inquire about the probability of it appearing 6 times, you can refer to the graph to find that it’s slightly more than 0.02 or 2%.
Understanding the Binomial Test
The binomial test is a statistical method used to determine if a dichotomous score follows a binomial probability distribution. Applying it to our example, you can rephrase the question in a way that allows for hypothesis testing:
Suppose you suspect that a die is biased in favor of showing the number 3. To investigate, you roll it 12 times (n = 12) and observe the number 3 (k = 5) on 5 occasions. You want to assess whether the die is indeed biased toward this outcome, considering the expected probability of observing a 3 is \(\frac{1}{6}\) or approximately 0.17. Formulating hypotheses, you have:
\(H_0: \pi \leq \frac{1}{6}\)
\(H_1: \pi > \frac{1}{6}\)
In this context, \(H_0\) represents the null hypothesis that the die is not biased, while \(H_1\) is the alternative hypothesis suggesting bias towards the number 3. Calculating the probability using the binomial distribution formula:
\[Pr(5;12,0.17)=Pr(X=5)=\frac{12!}{(12−5)! \times 5!} \times 0.17^5 \times (1−0.17)^{12−5} \approx 0.03\]
- Here, the calculated probability serves as the p-value for the significance test. Since the obtained p-value of 0.03 is less than the typical significance level of 0.05, you would reject the null hypothesis \(H_0\). This result suggests that the die is indeed biased toward showing the number 3, providing statistical evidence to support your suspicion;
- In practical terms, the binomial test empowers researchers and analysts to rigorously test hypotheses about binary outcomes, enhancing the credibility of their findings and aiding in data-driven decision-making;
- The binomial test is a vital statistical tool used to determine if observed data aligns with expectations based on a binomial probability distribution. In our example, it was employed to assess whether a die’s behavior deviated from randomness. By framing the research question as a hypothesis test, we could rigorously evaluate the die’s propensity to favor the number 3.
This statistical approach is not limited to dice; it has widespread applications in various fields. For instance, in pharmaceutical trials, it can determine if a new drug outperforms a placebo. In quality control, it can ascertain whether a manufacturing process meets defined standards. In essence, the binomial test plays a pivotal role in verifying hypotheses and making informed decisions based on empirical data.
Its versatility and ability to provide statistically sound conclusions make the binomial test an indispensable tool in the arsenal of statisticians, researchers, and decision-makers across diverse domains.
Performing the Binomial Test in Python (Example)
To execute the binomial test in Python, you can utilize the `binomtest()` function from the scipy library. Here’s a straightforward implementation:
- Step 1: Import the function.
```python
from scipy.stats import binomtest
```
- Step 2: Define the number of successes (k), the number of trials (n), and the expected probability of success (p).
```python
k = 5
n = 12
p = 0.17
```
- Step 3: Execute the binomial test in Python.
```python
res = binomtest(k, n, p)
print(res.pvalue)
```
You will obtain a p-value, which corresponds to the significance test’s result. In our case, it’s approximately 0.039, similar to the value calculated manually earlier. Note: By default, the test performed is a two-tailed test. If you are working with a one-tailed test scenario, please refer to the scipy documentation for this function.
Conclusion
In conclusion, this tutorial has provided a comprehensive understanding of the binomial distribution and its practical application through Python. It started by emphasizing the importance of having specific Python libraries, such as scipy, numpy, and matplotlib, installed to work with statistical distributions effectively. The step-by-step breakdown, complete with code snippets, made it accessible for readers to grasp and apply these concepts.
- The tutorial elucidated the fundamental aspects of the binomial distribution, elucidating how it models the probability of success and failure in a series of independent experiments. Moreover, it elucidated the calculation of probabilities and explained the significance of p-values in hypothesis testing. The visualization of the binomial distribution using matplotlib provided a visual representation of these probabilities, making it easier to comprehend;
- The inclusion of a practical example involving rolling a die multiple times and calculating the probability of a specific outcome further solidified the understanding of these statistical concepts. This example served as a real-world illustration of how the binomial distribution can be employed to make informed decisions based on data analysis;
- Furthermore, the tutorial demonstrated how to perform a binomial test in Python, a vital statistical technique for testing hypotheses related to binomial data. By providing clear instructions and code snippets, readers gained the knowledge and tools required to conduct their own statistical tests.
In essence, this tutorial not only conveyed the theoretical foundations of the binomial distribution but also equipped readers with practical skills in Python for data analysis and hypothesis testing. These skills are indispensable for professionals and researchers across diverse fields, enhancing their ability to draw meaningful insights from data and make informed decisions based on statistical evidence.
Average Rating