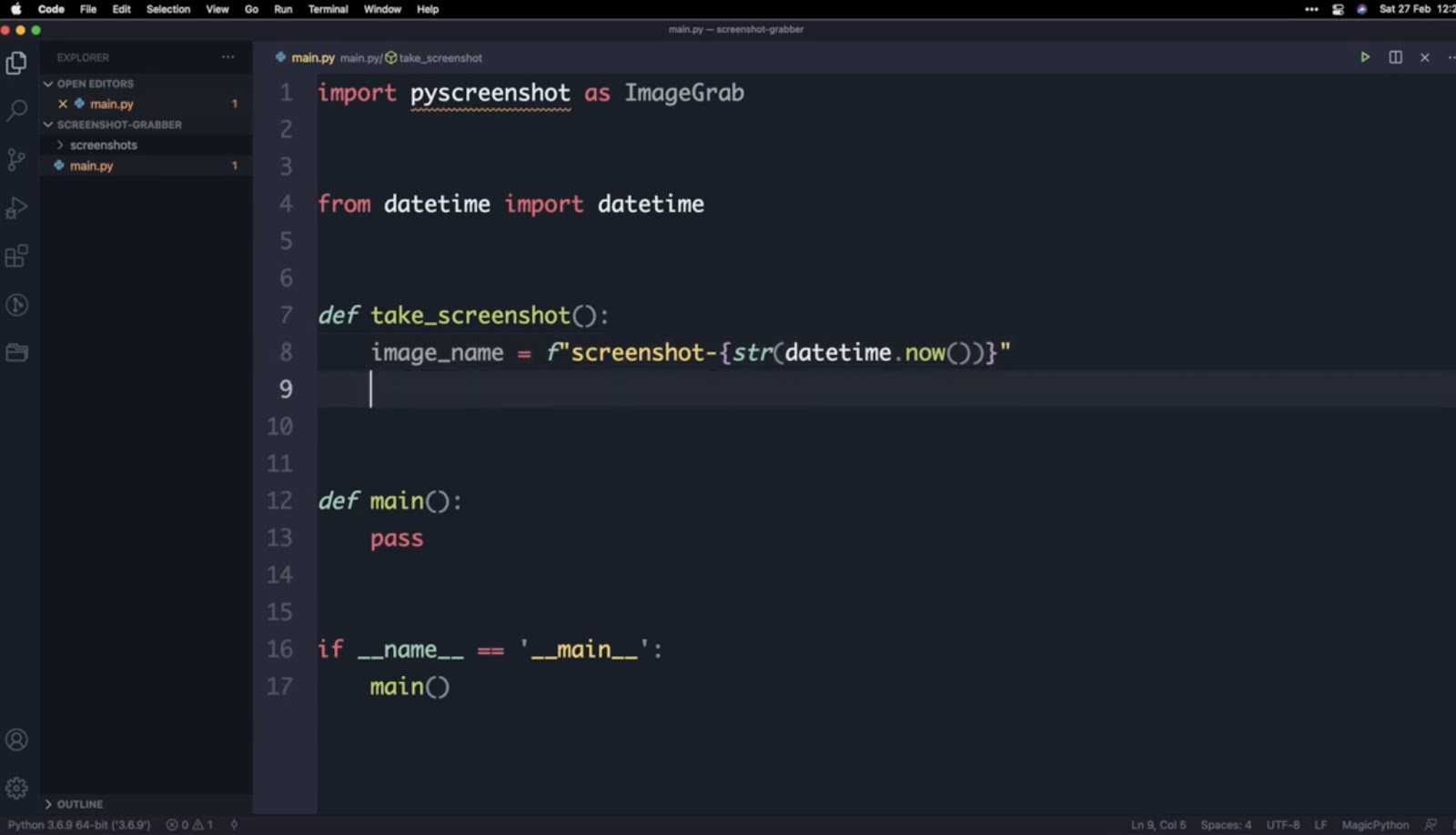
Python Screenshot: Capture and Save Code Screenshots
The process of capturing computer screenshots can often be a manual task, but it’s also possible to automate it using Python and various libraries. This narration explores the concept in the third person and restructures the information.
Automating Screenshots with Python
Capturing screenshots on a computer is a common task, and both Windows and Mac provide built-in methods for this purpose. However, there are scenarios where automating the process becomes essential, such as taking screenshots at regular intervals. Doing this manually would be time-consuming and impractical. Fortunately, Python offers a solution with the support of several libraries.
Using pyautogui for Fast Screen Capture
For those working with a single monitor or knowing specific screen coordinates, pyautogui is a swift and convenient choice. The following steps demonstrate how to use it:
- Import pyautogui:
Import the pyautogui library into your Python script.
```python
import pyautogui
```
- Capture the Screen:
Utilize the `.screenshot()` method to capture the screen, and save the result with a chosen filename.
```python
myscreen = pyautogui.screenshot()
myscreen.save('myscreen.png')
```
This code will generate a .png file in your script’s directory.
Taking Screenshots with PIL in Python
PIL (Python Imaging Library) is a renowned Python library for image processing. It also offers screenshot functionality. Here’s how to use it:
- Import PIL’s ImageGrab Module:
Start by importing the ImageGrab module from the PIL library.
```python
from PIL import ImageGrab
```
- Capture the Screen:
Similar to pyautogui, use the `.grab()` method to capture the screen and save the image.
```python
myscreen = ImageGrab.grab()
myscreen.save('myscreen_PIL.png')
```
This will produce a .png file in your script’s directory.
Screenshot Capture for Multiple Monitors with mss in Python
If you’re dealing with multiple monitors, the mss library in Python simplifies the process. Follow these steps:
- Import mss:
Begin by importing the mss library.
```python
from mss import mss
```
- Capture Screens:
To capture the current working screen, use `mss().shot()`. For multiple screens, loop through and specify the monitor using the `mon` parameter.
```python
for i in range(1, 3):
mss().shot(mon=i)
```
Running this code will generate two .png files in your directory.
Conclusion
In this comprehensive guide, the power of Python for automating the process of capturing computer screenshots has been unveiled. While both Windows and Mac offer built-in screenshot capabilities, Python takes it a step further by allowing users to automate this task effortlessly.
- The journey began with **pyautogui**, a library that proves to be the fastest and most convenient choice, especially for single-monitor users or those with specific screen coordinates in mind. With just a few lines of code, users can effortlessly take full-screen or targeted screenshots;
- Next, we explored **PIL**, the Python Imaging Library, known for its image processing prowess. Surprisingly, it also provides a straightforward solution for capturing screens, making it a versatile tool for image-related tasks;
- For those with multiple monitors, the **mss** library emerged as the hero of the story. With its capabilities, users can capture screens across all displays with ease, providing a valuable solution for a more complex setup.
In conclusion, Python empowers individuals to streamline the screenshot capture process, whether for personal use or in automation scenarios. These libraries offer a range of solutions, from quick and simple captures to more intricate multi-monitor setups. With Python’s versatility and these libraries at your disposal, you’re well-equipped to harness the potential of automated screenshot capture in your projects and workflows.
Average Rating