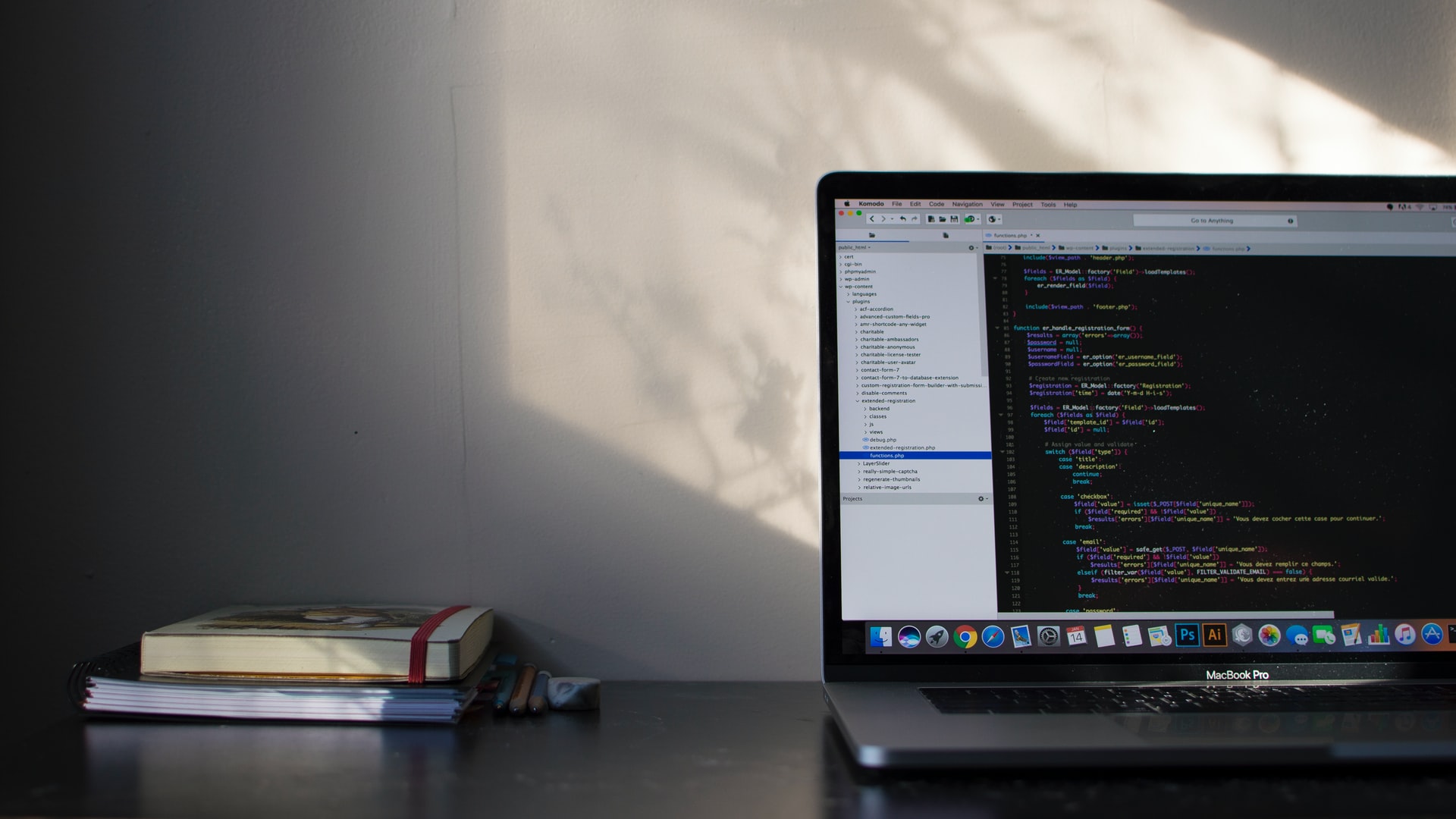
Ruby: Classes
OOP is a big topic in Ruby with a million different features of its own. Right now we’re not ready to cover it thoroughly, but at the very least you need to know some basic constructs that are used extensively. First of all, we’re talking about classes. Look at date creation in Ruby:
# Naming classes in Ruby follows the CamelCase style,
# which distinguishes them from functions/methods and variables
# Method calls below
d = Date.today
d.next # returns the next day object
# Creates the date for today and the current time
time = Time.new
time.thursday? # false
Time is a class. And the entry itself means: call the new() method of the class. In Ruby, new is not a separate operator, but an ordinary method. Of course, it’s not exactly a regular method, but syntactically it is no different from regular methods. This syntax is there for a reason, and you’ll see why below. At the same time it makes it easier to make chains “in place”: Time.new.to_i (returns unixtimestamp). More examples
# Creates a date for 00:00 11-12-2022
Time.new 2022, 12, 11
As we remember, the constructor does not belong to objects, but to the class itself, so it is called on the class. But since it is a method, what is a class? Take a look at a couple more examples:
# now, not new
time1 = time.now
time2 = Time.utc # returns the time object in utc
Here, depending on your background, the answers may vary. Someone in PHP will say that now is a static method, someone else will say that maybe there are no classes, but only objects, like in JavaScript. In Ruby there are no static properties and methods, and there are real classes. But classes are objects that have their own methods and classes.
Time.methods.count # 126
Time.class # the Time class is Class
Time.class.class # and Class class is Class :D
Time.superclass # and the class also has a parent Object
Time.superclass.class # which class is Class
Time.superclass.superclass # and the parent is BasicObject
# And we're not even talking about object classes
# And you can also create a class
Class.new ... # since a class is not only an object but also a class
That is, the phrase in Ruby “everything is an object” should be taken almost literally, but it is very different from the same JavaScript, where by object we mean a specific data type object. Ruby’s object model is closest to the SmallTalk language. At first glance it’s intimidating, but over time you don’t just get used to it, it becomes intuitive.
What to do with it at all? Nothing for now. Knowing how to work with classes and how to construct them will be needed when interacting with frameworks and libraries. This topic is covered at the very end of the course, but for now, a little example from Rails:
# Just a show-and-tell, you don't have to understand this code
class User < ApplicationRecord # < - inheritance
# this is a method call right in the class definition
validates :email, presence: true, uniqueness: true
# and these are method calls
has_many :companies
belongs_to :company
def to_s
# this/self are not needed
"#{first_name} #{last_name}"
end
end
Average Rating