Convert Python DOCX to PDF with PDFify
This narrative will guide you through the process of converting PDF files to Microsoft Word docx format and vice versa using Python. It will cover the use of two essential libraries: pdf2docx and docx2pdf.
- Document format conversions are a common need in various professional and academic scenarios. Whether you want to extract content from a PDF for further editing or create PDFs from your Word documents, Python provides a powerful and efficient solution. This guide ensures that you have the tools and knowledge to seamlessly perform these conversions;
- The pdf2docx library simplifies the extraction of tables, text, and content from PDF files, allowing you to work with the data within Microsoft Word. Conversely, docx2pdf streamlines the process of generating PDFs from your docx documents, ensuring compatibility and easy sharing.
With clear instructions, code examples, and sample files provided, you’ll be equipped to tackle document format conversions confidently. Whether you’re a seasoned Python developer or just starting, this guide offers a practical approach to handling document formats efficiently and effectively.
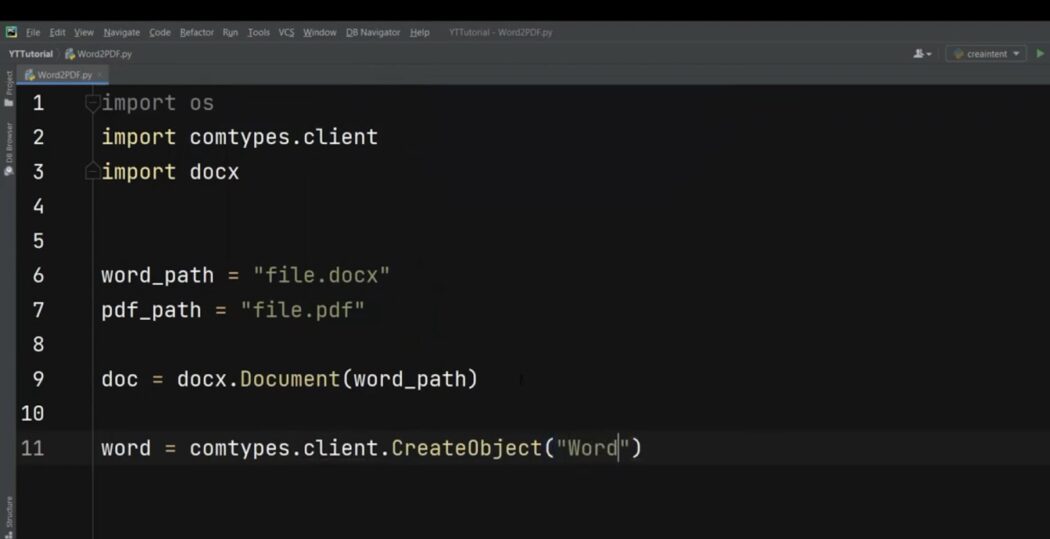
Prerequisites
Before diving into the conversion process, make sure you have these Python libraries installed:
pdf2docx
```shell
pip install pdf2docx
```
docx2pdf
```shell
pip install docx2pdf
```
Sample Files
To follow along with this tutorial, you’ll need sample files to practice the PDF-to-docx and docx-to-PDF conversions. Here’s how you can get started:
- For the PDF-to-docx conversion, you will require a sample PDF file. You can download the example file by clicking on the following link: [sample.pdf](sample.pdf);
- Once you’ve downloaded the sample PDF file, it’s important to place it in the same directory or folder where your Python script is located. This ensures that Python can easily access the PDF file when you run the conversion code;
- Similarly, for the docx-to-PDF conversion, you’ll need a sample docx file. You can obtain this sample docx file by clicking on the following link: [input.docx](input.docx);
- Just like with the PDF file, make sure to save the sample docx file in the same directory as your Python script. This organization simplifies the conversion process, as Python will be able to locate and convert the docx file without any issues.
Having these sample files at your disposal allows you to experiment with the conversion processes described in the tutorial. You can confidently follow the provided code examples, knowing that you have the necessary files ready for conversion. This hands-on approach ensures that you gain practical experience in working with PDF and docx file formats using Python.
Converting PDF to Docx
Using the pdf2docx library, you can achieve this conversion effortlessly. Below are two methods:
- Method 1:
```python
from pdf2docx import Converter
pdf_file = 'sample.pdf'
docx_file = 'sample.docx'
cv = Converter(pdf_file)
cv.convert(docx_file)
cv.close()
```
Method 2:
```python
from pdf2docx import parse
pdf_file = 'sample.pdf'
docx_file = 'sample.docx'
parse(pdf_file, docx_file)
```
For both methods, the resulting `sample.docx` will appear in the same directory.
Converting a Single Page
To convert a specific page from a PDF file, follow these steps:
```python
from pdf2docx import Converter
pdf_file = 'sample.pdf'
docx_file = 'sample.docx'
pages_list = [0] # Index of the page you want to convert
cv = Converter(pdf_file)
cv.convert(docx_file, pages=pages_list)
cv.close()
```
Converting Docx to PDF
Now, let’s dive into the process of converting docx files to PDF using the docx2pdf library. This library simplifies the task, allowing you to generate PDFs from your docx documents with ease.
To begin the conversion, you can use the following Python code snippet:
```python
from docx2pdf import convert
docx_file = 'input.docx'
pdf_file = 'output.pdf'
convert(docx_file, pdf_file)
```
In this code:
- We import the `convert` function from the `docx2pdf` library;
- You need to specify the path to your input docx file, which in this case is `’input.docx’`. This is the document you want to convert to PDF;
- Next, specify the path where you want to save the resulting PDF file. In this example, it’s set to `’output.pdf’`.
Once you run this script, the docx file specified as input will be converted into a PDF document. You will find the generated PDF file in the directory where your Python script is located, under the name you provided, which in this case is `’output.pdf’`.
The docx2pdf library streamlines this conversion process, making it quick and straightforward. Whether you need to create PDF versions of your documents for sharing or archiving, this library is a valuable tool in your Python toolkit.
Conclusion
In this comprehensive guide, we’ve explored the art of converting PDF files to Microsoft Word docx format and reciprocally transforming docx files into PDFs using Python. The process is made simpler thanks to two crucial libraries: pdf2docx and docx2pdf.
- Before embarking on this journey, it’s imperative to ensure you have the necessary prerequisites installed. Specifically, the pdf2docx and docx2pdf libraries are vital for these operations. If not already installed, the provided commands will swiftly get you up to speed. Furthermore, we’ve made the journey practical by providing sample files for you to experiment with. These sample files enable hands-on experience, and we’ve included clear instructions on how to set up your working environment;
- Converting PDFs to docx format is remarkably straightforward with the pdf2docx library. We’ve illustrated two methods to cater to various preferences. Whether you choose Method 1 or Method 2, the end result is a conveniently converted docx file ready for editing. Additionally, we’ve delved into the process of converting a specific page from a PDF file, offering even more flexibility in your PDF-to-docx conversions;
- Conversely, when the need arises to transform docx files into PDFs, the docx2pdf library comes to the rescue. With just a few lines of Python code, you can convert your docx documents into PDF format effortlessly.
In conclusion, Python proves to be a versatile tool for managing document formats. The libraries mentioned here make complex conversions accessible to all, and with this newfound knowledge, you can confidently navigate the world of document format transformations. We encourage you to explore the official documentation of these libraries to unlock their full potential and embark on more document-related adventures with Python at your side. Happy converting!
Average Rating