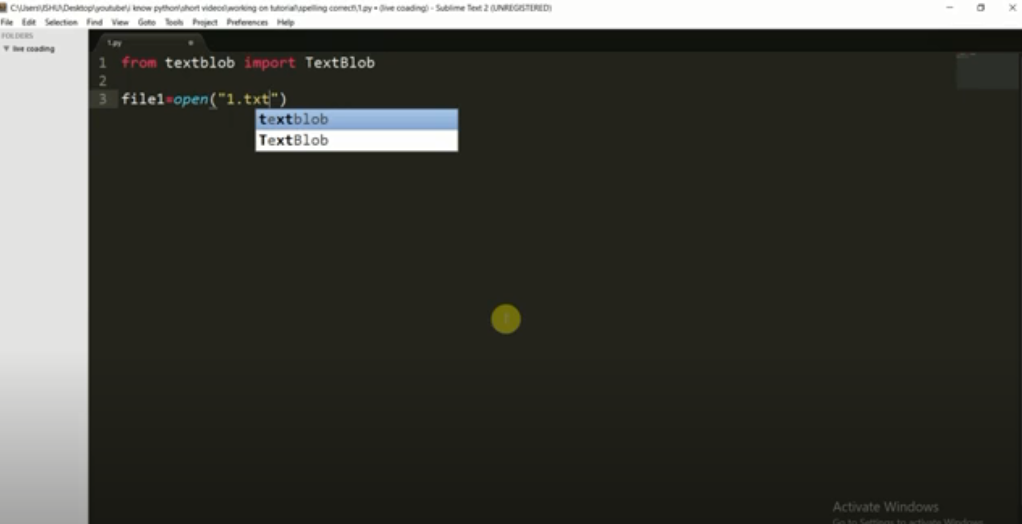
Spell Checker Program in Python
While spell checkers are handy tools, they often don’t meet the demands of real-world scenarios. Practical use cases frequently require software that can not only identify spelling mistakes but also automatically rectify them.
In this guide, we will explore how Python can be employed to fix spelling inaccuracies in both individual words and complete sentences. Being able to automate the correction of text is an indispensable asset for enhancing the textual quality across different applications.
Steps to Autocorrect Spelling in Single Words
We’ll look at how to use Python to correct spelling errors in both words and sentences. This skill is especially valuable for improving the quality of text in various applications.
Step 1: Import Necessary Libraries
The initial step involves importing the essential modules. For this purpose, the ‘Word’ class from the ‘textblob’ library is indispensable, as it provides a variety of methods for spell correction.
from textblob import Word
Step 2: Choose a Word for Spelling Rectification
As the next step, pinpoint a word that necessitates spelling rectification. In our example, let’s consider the misspelled word ‘appple’.
incorrect_word = Word('appple')
Step 3: Apply Spelling Rectification to the Chosen Word
Proceed to execute the spelling correction on the selected word and then display the corrected version.
corrected_output = incorrect_word.correct() print(corrected_output)
Upon running, this should display ‘apple’ as the corrected output.
Building a Complete Word Spelling Autocorrector
By integrating these steps and augmenting them with additional features, a comprehensive program for word spelling correction can be created.
from textblob import Word def autocorrect_word(incorrect_word): incorrect_word = Word(incorrect_word) corrected_output = incorrect_word.correct() print(corrected_output) autocorrect_word('appple')
Executing this program using ‘appple’ should return the corrected word, ‘apple’.
Steps to Autocorrect Spelling in Sentences
Step 1: Import the Necessary Libraries
For sentence spelling correction, the ‘TextBlob’ class from the ‘textblob’ library is crucial.
from textblob import TextBlob
Step 2: Select a Sentence for Spelling Rectification
Identify a sentence that contains spelling inaccuracies. For illustration, let’s use the sentence ‘A sentencee to checkk!’, which has obvious errors.
incorrect_sentence = TextBlob('A sentencee to checkk!')
Step 3: Execute Spelling Rectification on the Sentence
Apply the spelling correction on the selected sentence and display the rectified text.
corrected_sentence_output = incorrect_sentence.correct() print(corrected_sentence_output)
This should return the corrected sentence: ‘Sentence to check!’ when executed.
Program for Correcting Spelling in Sentences
To create a program for correcting spelling in sentences using Python, combine the steps above and add functionality.
def correct_sentence_spelling(sentence):
sentence = TextBlob(sentence)
result = sentence.correct()
print(result)
correct_sentence_spelling('A sentencee to checkk!')
Running this program with the sample sentence ‘A sentencee to checkk!’ should return ‘A sentence to checkk!’.
What is the Python library to check typos?
A Python library often used to check and correct typos in text is called “TextBlob”. Below is more information about TextBlob:
TextBlob
TextBlob is a popular Python text processing library that offers a wide range of natural language processing (NLP) features, including sentiment analysis, part-of-speech tagging, translation, and, importantly, spell-checking and correction;
- Spell Checker: TextBlob provides a simple and effective way to identify and correct spelling errors in words and sentences. It utilizes language models and dictionaries, offering context-aware corrections;
- Ease of Use: One of the key advantages of TextBlob is its user-friendly and intuitive API. It is easy to install and use, making it suitable for both beginners and experienced developers;
- Multi-language correction: TextBlob supports multiple languages, making it versatile for checking and correcting spelling in different contexts and languages;
- Integration: TextBlob can be easily integrated into Python applications and scripts, making it a valuable tool for text processing and data analysis projects;
- Extensibility: TextBlob can be extended with custom models and vocabularies, allowing it to be customized for specific domains or languages;
- Community Support: TextBlob has an active community of users and developers, which means you can find documentation, tutorials, and support online.
Here’s a simple example of using TextBlob to check and correct spelling in Python:
from textblob import Word
# Create a Word object with the misspelled word in it
word = Word('appple')
# Correct the spelling
corrected_word = word.correct()
print(corrected_word) # Output: 'apple'
In addition to correcting individual words, TextBlob can correct spelling in whole sentences using the TextBlob class. This is a versatile and valuable library for text-processing tasks, especially when it comes to fixing typos and improving text quality.
Conclusion
In this extensive exploration of Python’s spelling correction capabilities, we discovered a valuable tool in the form of the TextBlob library. Whether you have to deal with typos in single words or entire sentences, TextBlob simplifies the process and improves the overall quality of your text.
By following step-by-step guides, you will acquire the skills to not only identify but also correct spelling errors without much effort. TextBlob’s versatility, multi-language support, and convenient API make it an indispensable assistant in any text processing or data analysis project.
With the help of TextBlob, you can increase the accuracy and professionalism of your work with text. If you develop applications, conduct research, or simply strive for flawless written communication, the TextBlob library in Python will be your optimal solution for efficient typo detection and correction.
Average Rating