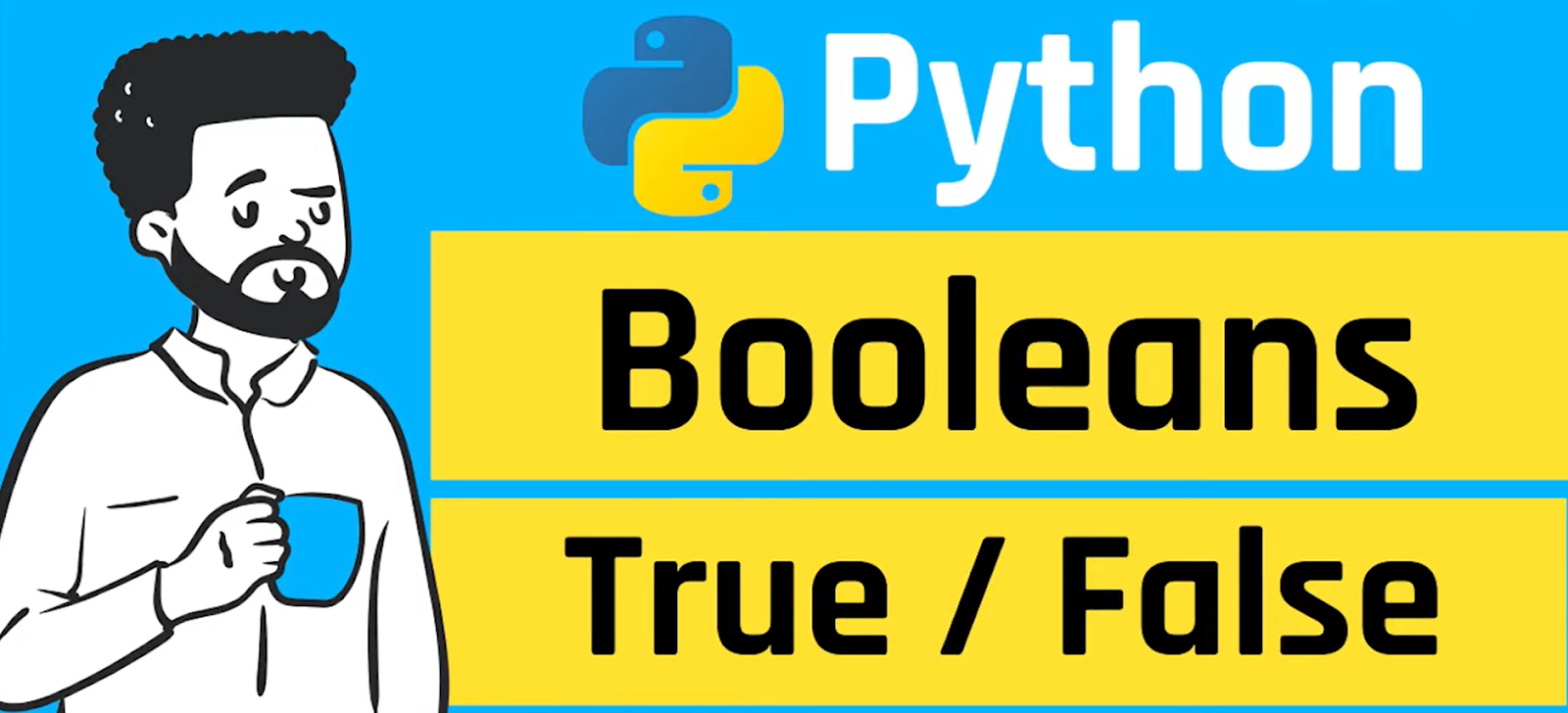
Python’s Boolean Type: Comprehensive Breakdown
In this comprehensive article, we will delve into the world of Python’s boolean data type, shedding light on its fundamental concepts, practical applications, and various nuances.
In Python, the boolean data type is categorized as a subtype of the numeric data type. It serves as a binary representation, capable of holding one of two values: True or False, which can equivalently be expressed as integers 1 and 0.
Familiarizing yourself with the various data types in any programming language is a fundamental step towards comprehending code and applications.
The boolean data type plays a pivotal role in numerous domains, including mathematics, statistics, data science, and machine learning, where Python is frequently employed as the programming language of choice. Its versatility and efficiency make it a cornerstone of many Python-based solutions within these fields.
Creating Booleans in Python
In Python, generating boolean values is a straightforward process that offers flexibility for various programming needs. You can create booleans in three distinct ways, each suited to different scenarios.
- Setting a Variable’s Value to True or False;
- Utilizing Python’s bool() Constructor;
- Employing Boolean Expressions.
Assigning True or False Values to Variables in Python
In Python, you can effortlessly assign True or False values to variables, creating boolean variables to represent binary conditions. Let’s walk through an example of how to do this:
- True;
- False.
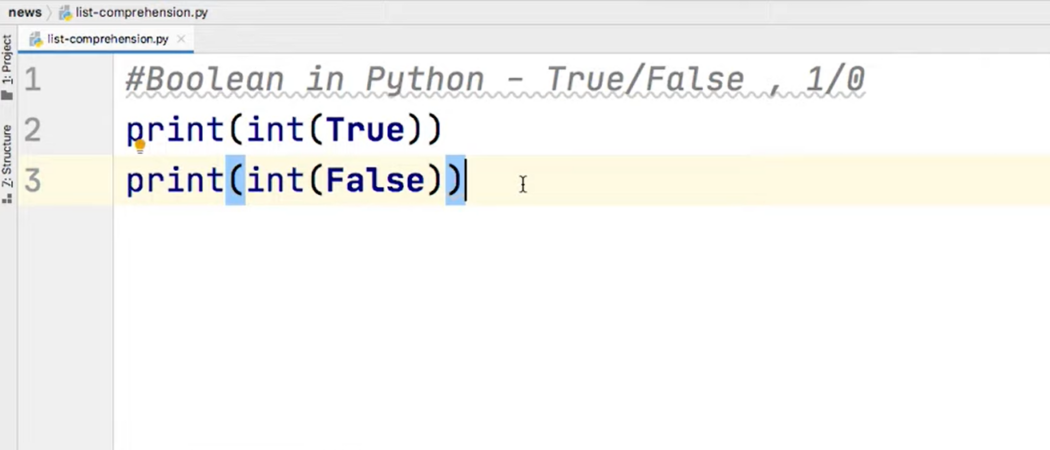
Creating Booleans in Python Using the bool() Constructor
In Python, you have the option to create boolean values using the bool() constructor. This constructor serves as a versatile tool for generating booleans based on different input conditions.
When you use the bool(argument) constructor, it evaluates the specified argument and returns its corresponding boolean value. Here’s what you can expect:
- It returns True if the argument is any number (except zero), the boolean value True, or a non-empty string;
- Conversely, it returns False if the argument is empty, the boolean value False, or None.
Now, let’s explore some practical examples of using the bool() constructor with arguments that result in True values:
#Number
t1 = bool(5)
#True
t2 = bool(True)
#String
t3 = bool('Python')
#Print variables
print(t1, t2, t3)
and you will get:
True True True
Now, let’s delve into a selection of examples where the bool() constructor yields False values:
#Empty
f1 = bool()
#Empty list
f2 = bool([])
#False
f3 = bool(False)
#None
f4 = bool(None)
#Zero
f5 = bool(0)
#Print variables
print(f1, f2, f3, f4, f5)
and you will get:
False False False False False
Creating Booleans with Boolean Expressions in Python
In Python, another way to generate boolean values is through the use of boolean expressions. These expressions are essentially evaluations that result in either a True or False outcome.
For instance, consider the comparison of two numbers:
For instance, consider the comparison of two numbers:
#Create two numbers x and y
x = 5
y = 3
#Evaluate using boolean expression
is_x_greater_than_y = x > y
#Print boolean
print(is_x_greater_than_y)
and you will get:
True
Below are the comparison operations available for evaluating a boolean expression:
Operation | Result (boolean) |
---|---|
x > y | x is greater than y |
x >= y | x is greater than or equal to y |
x < y | x is less than y |
x <= y | x is less than or equal to y |
x == y | x is equal to y |
x != y | x is not equal to y |
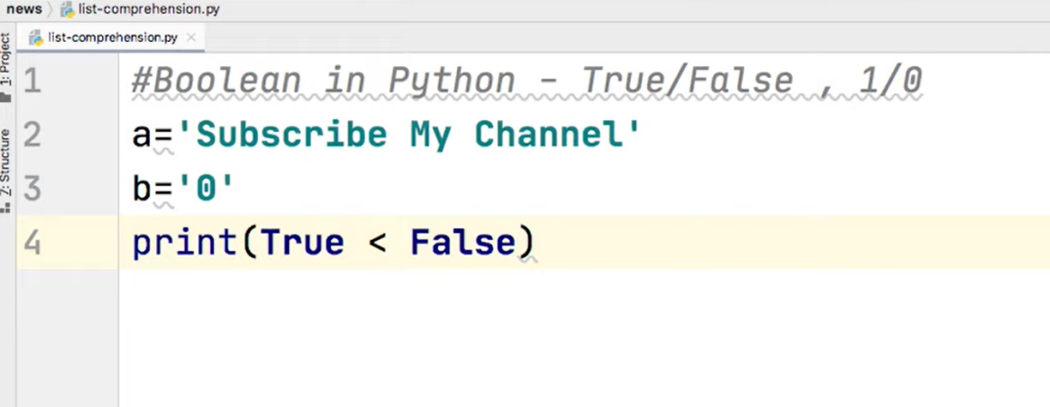
Leveraging Booleans in Python’s Control Structures
In Python, booleans play a pivotal role in control structures that dictate the program’s execution flow. One of the most straightforward examples of this application is evident in Python’s `if` statements:
#Create two numbers x and y
x = 5
y = 3
#Create a control structure
if x > y:
print('x is greater than y')
else:
print('x is not greater than y')
and you will get:
x is greater than y
Conclusion
In this comprehensive article, we have delved deep into the world of Python’s boolean data type, examining its multifaceted applications, from its role in boolean expressions to its integral place within control structures. By gaining a thorough understanding of booleans in Python, you are now well-equipped to employ this fundamental data type effectively in your programming endeavors, enabling you to write more efficient and responsive code. As you continue your Python journey, remember that mastering booleans is just one step towards becoming a proficient Python programmer, and the knowledge you’ve acquired here will serve as a solid foundation for your future endeavors in the world of programming.
Average Rating