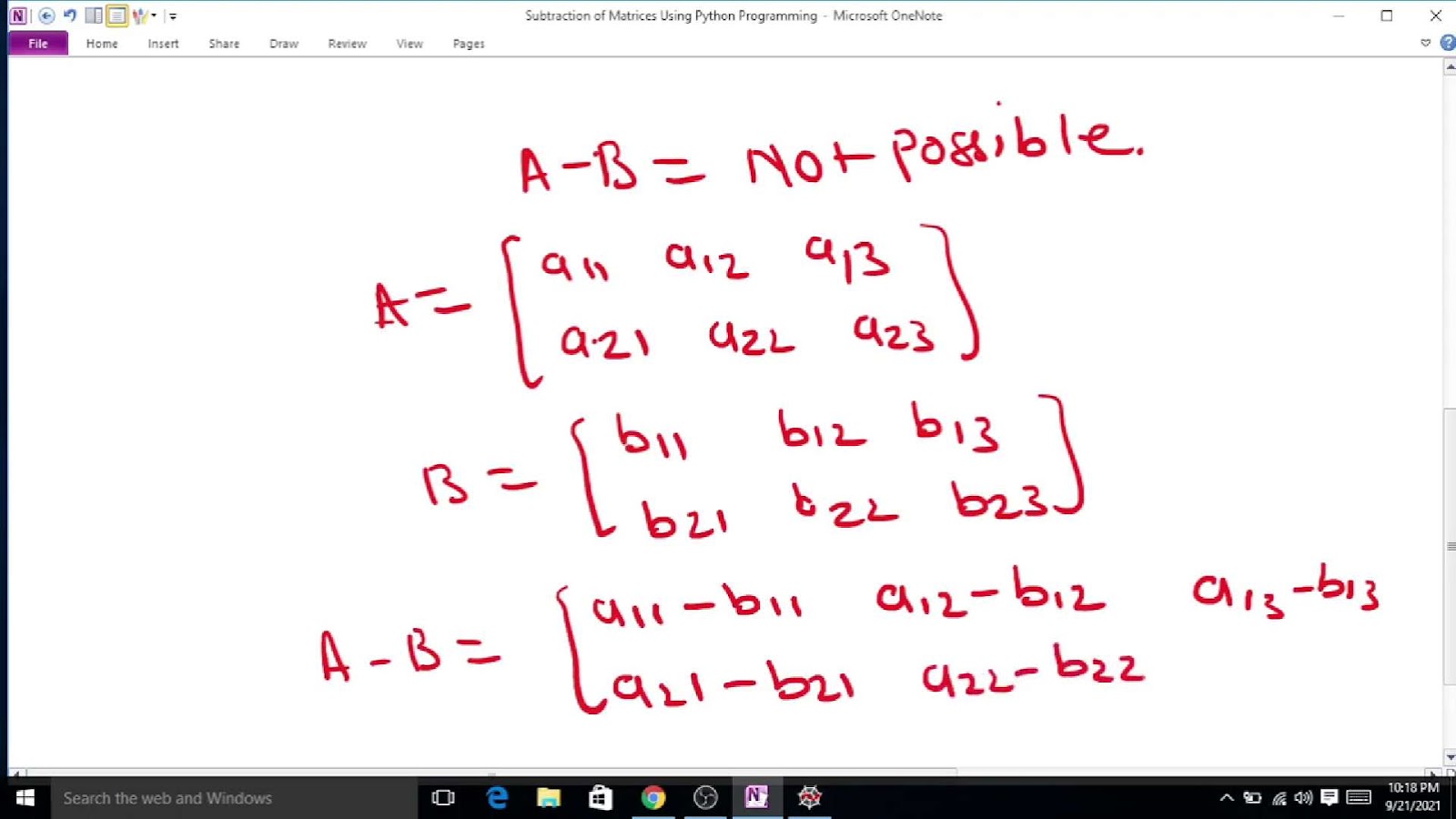
Performing Matrix Subtraction in Python
Matrices are fundamental mathematical constructs with widespread applications in fields ranging from computer science and physics to engineering and data analysis. They serve as powerful tools for organizing and manipulating data, making them indispensable in various computational tasks. In this article, we will delve into the world of matrix operations, focusing specifically on matrix addition and subtraction, two fundamental operations that form the backbone of linear algebra and numerical computing.
Our journey will take us through the essential steps and intuitive concepts behind matrix addition and subtraction, with a particular emphasis on their practical implementation in Python. Whether you’re a seasoned programmer or just starting your journey in the realm of mathematics and coding, this comprehensive guide will equip you with the knowledge and skills needed to perform these operations confidently.
Matrix Subtraction: A Comprehensive Guide
Matrix subtraction might seem like a daunting task for those new to the concept, but with the right intuition and understanding, it becomes a straightforward process. This guide delves deep into the mechanics of it, providing simple examples and also demonstrating how Python, a popular programming language, simplifies the task even further.
A Brief Overview
- Core Concept: At its essence, matrix subtraction is quite similar to matrix addition. It involves taking two matrices of the same dimension and subtracting the elements of one matrix from the corresponding elements of the other matrix;
- Simple Examples: The examples provided in this guide are designed to be straightforward, ensuring that readers can grasp the fundamental principles without the need for complex calculations or tools.
Advancing Beyond the Basics
Even though this guide starts with basic examples, the techniques discussed here can be effectively applied to more intricate matrix subtraction problems. By building a strong foundation, readers can confidently tackle even the most challenging matrix operations.
Python and Matrix Operations: A Perfect Duo
Python, a versatile programming language, has several libraries that make matrix operations, including subtraction, a breeze. One of the most prominent libraries for this purpose is numpy.
Benefits of using it:
- Efficiency: Matrix operations can be performed with just a few lines of code;
- Flexibility: Python’s libraries can handle matrices of varying sizes and complexities;
- Visualization: With the right tools, it can also be used to visualize matrices, aiding in better understanding and interpretation.
Setting Up Your Python Environment
To ensure a smooth experience, it’s essential to have the numpy library installed. This section guides readers through the installation process.
Steps to Install numpy:
- Windows Users: Open the “Command Prompt.”;
- Type in the following command and press enter:
pip install numpy
Wait for the installation process to complete.
Tips for a Successful Installation:
- Ensure your Python and pip versions are up-to-date;
- If you encounter any errors, consult Python’s official documentation or forums for troubleshooting.
Understanding Matrix Subtraction
Matrix subtraction is a straightforward operation applied to matrices. However, for this subtraction to occur, there’s a crucial criterion to be met: both matrices must be of identical dimensions. This means that if one matrix is 2×2, the other should also be 2×2; for a 3×3 matrix, its counterpart should also be 3×3, and so forth.
A Practical Illustration
Imagine a scenario where two farmers each possess specific quantities of apples and grapes in their storage facilities. Visualizing this situation can be achieved by using a table:
Items | Farmer 1 | Farmer 2 |
---|---|---|
Apples | 3 | 2 |
Grapes | 7 | 5 |
This table’s representation in the realm of matrices would be:
A =3275
Suppose both farmers decide to sell some of their stock at a market. The number of fruits they sold can be similarly tabulated:
Items | Farmer 1 | Farmer 2 |
---|---|---|
Apples | 2 | 1 |
Grapes | 1 | 3 |
This data in matrix form becomes:
B = 2113
After the day’s sales, the farmers decide to take stock of their remaining inventory. The simplest way to find out the residual stock is by subtracting the quantities of the sold fruits from the initial stock.
Resulting inventory:
Items | Farmer 1 | Farmer 2 |
---|---|---|
Apples | 1 | 1 |
Grapes | 6 | 2 |
Performing this subtraction with matrices, we get:
C = A – B =
3275 -2113
= 1162
This result C matches perfectly with the tabulated inventory data.
Generalization to Larger Matrices
Matrix subtraction isn’t confined to 2×2 matrices. For any m×n matrix, the subtraction operates in a cell-by-cell manner. Represented mathematically:
If A = a(ij) and B =b(ij) where i = 1,…,m and j = 1,…,n, then the result of the subtraction, C = A – B, will have its elements c_(ij) such that: c_(ij) = a_(ij) – b_(ij) for every i and j.
In simpler terms, each element in matrix A is subtracted from the corresponding element in matrix B to yield the resultant matrix C.
Matrix Subtraction Using Python and Numpy
Matrix operations are a core concept in linear algebra and have extensive applications in various domains, ranging from data analysis to computer graphics. Python, being a versatile programming language, offers an array of tools to manipulate and operate on matrices. One of the most powerful libraries for this purpose is NumPy.
Integrating NumPy for Matrix Operations
To get started with matrix operations in Python, it’s crucial to have the NumPy library, which provides numerous functionalities for matrix arithmetic. Begin by integrating this library into the script:
import numpy as np
NumPy not only makes matrix operations intuitive but also optimizes them for better performance.
Constructing Matrices in Python
In Python, matrices can be visualized as multi-dimensional arrays. When it comes to matrix subtraction, it’s paramount that the matrices have identical dimensions. This ensures that each element in one matrix has a corresponding element in the other matrix to pair with during the subtraction process.
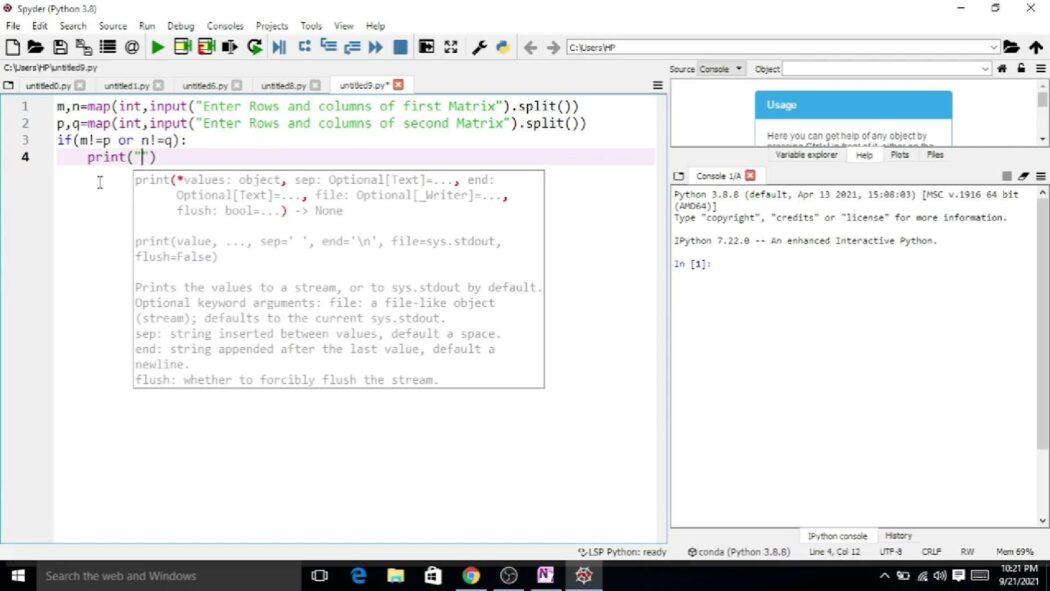
For this demonstration, consider two 2×2 matrices:
A = np.array([[3, 2],
[7, 5]])
B = np.array([[2, 1],
[1, 3]])
These matrices consist of rows and columns of numbers that can be manipulated using NumPy’s functions.
Performing Matrix Subtraction
Matrix subtraction in NumPy is straightforward, thanks to the subtract() function. This function processes the matrices element-wise, subtracting corresponding elements from each matrix.
Execute the subtraction using the following code:
C = np.subtract(A, B)
print(C)
Upon executing the above, the output will be:
[[1 1]
[6 2]]
This result matches what would be obtained by manual calculations. With NumPy, the complexities of matrix arithmetic get abstracted, enabling users to focus on higher-level operations and applications.
Conclusion
In this comprehensive article, we delved into the intricacies of matrix subtraction, unraveling its intuitive essence and elucidating the meticulous steps involved. Furthermore, we exemplified these concepts through a series of fully-realized illustrative instances, skillfully implemented with the Python programming language.
Should you harbor inquiries or harbor a penchant for proffering constructive edits, we cordially invite you to share your thoughts and insights in the comments section below.
Average Rating