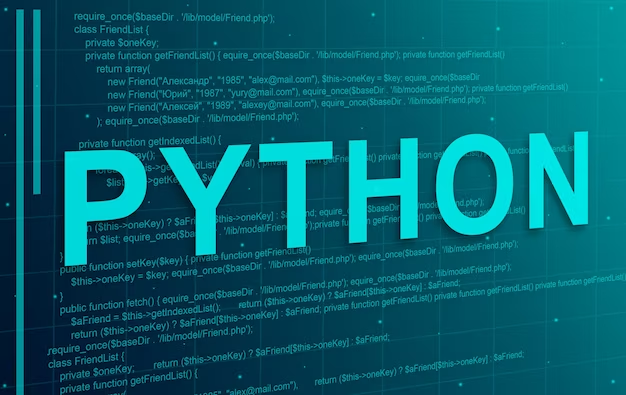
Everything About Python Dictionary Data Structure
In the realm of Python programming, the dictionary data structure is a versatile and indispensable tool. It allows developers to store and manage data efficiently, providing a bridge between keys and values. This comprehensive guide will take you on a journey through everything you need to know about Python dictionaries. From the basics to advanced techniques, we’ll explore its functions, use cases, and best practices, ensuring you have a solid grasp of this essential component in Python programming.
What is a Python Dictionary?
A Python dictionary is an unordered collection of data stored in a key-value pair format. Each key is unique, acting as an identifier for the associated value. Unlike other data structures, such as lists and tuples, dictionaries are highly efficient for retrieving and manipulating data based on these keys.
Dictionaries are enclosed in curly braces {} and consist of key-value pairs separated by colons. For example:

In this example, “name”, “age”, and “city” are keys, while “John”, 30, and “New York” are their respective values.
Creating and Initializing Dictionaries
Creating a dictionary in Python is straightforward. You can initialize an empty dictionary or populate it with key-value pairs during initialization.
Initializing an Empty Dictionary

Initializing a Dictionary with Key-Value Pairs

Accessing Dictionary Elements
Accessing elements in a Python dictionary is done by referring to the key associated with the value you want to retrieve. For instance, if you want to access the “name” value in my_dict, you can do so as follows:

Modifying Dictionary Elements
Dictionaries in Python are mutable, meaning you can change their values after creation. To modify a dictionary element, simply assign a new value to its corresponding key.

Dictionary Methods
Python provides a range of built-in methods to perform various operations on dictionaries. These methods simplify tasks such as adding, removing, or manipulating key-value pairs.
Here are some commonly used dictionary methods:
dict.keys()
This method returns a list of all the keys in the dictionary.
dict.values()
It returns a list of all the values in the dictionary.
dict.items()
This method returns a list of key-value pairs in the dictionary.
Iterating Over a Dictionary
Python offers several ways to iterate through a dictionary. You can loop through the keys, values, or both simultaneously using the items() method.
Iterating Through Keys

Iterating Through Values

Iterating Through Key-Value Pairs

Nested Dictionaries
Python dictionaries can be nested within one another. This allows you to create more complex data structures. For example:
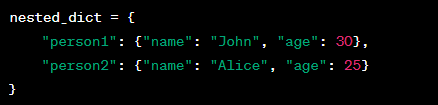
Dictionary Comprehensions
Similar to list comprehensions, Python allows you to create dictionaries using dictionary comprehensions. These concise expressions make dictionary creation elegant and efficient.

Common Use Cases
Python dictionaries find applications in various domains, thanks to their flexibility and efficiency. Here are some common use cases:
- Data Storage: Dictionaries are ideal for storing data with unique identifiers;
- Configuration Settings: They are used to store configuration settings for applications;
- Counting: Dictionaries are helpful for counting occurrences of items in a dataset;
- Caching: They are employed for caching frequently accessed data to improve performance.
Best Practices for Using Python Dictionaries
To make the most of Python dictionaries, consider the following best practices:
- Choose Meaningful Keys: Use descriptive keys to make your code more readable;
- Avoid Changing Keys: It’s recommended to keep dictionary keys immutable;
- Use Dictionary Methods: Leverage built-in methods to perform operations efficiently;
- Error Handling: Check if a key exists before accessing it to avoid KeyError exceptions;
- Keep Dictionaries Simple: Avoid deeply nested dictionaries for better code maintainability.
Advanced Dictionary Techniques
Dictionary Merging
Sometimes, you may need to combine two dictionaries to consolidate data. Python provides a straightforward way to merge dictionaries using the update() method or the {**dict1, **dict2} syntax. This technique proves handy when dealing with configuration settings or data from multiple sources.
Dictionary Views
Python 3.0 introduced dictionary views, which are dynamic representations of dictionary keys, values, or key-value pairs. These views provide an up-to-date reflection of the dictionary’s contents. You can use keys(), values(), and items() methods to access these views. They are particularly useful for monitoring and manipulating dictionary data in real-time.
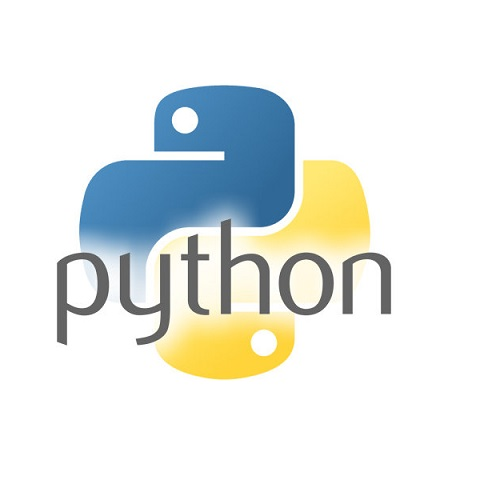
Performance Considerations
Dictionary vs. Lists
While dictionaries offer efficient key-based data retrieval, lists excel in sequential access. If you need to maintain order or perform index-based operations, lists might be a better choice. Consider your specific use case to determine which data structure suits your needs.
Memory Usage
Keep in mind that dictionaries consume more memory than lists or sets due to the overhead of storing key-value pairs. If memory optimization is crucial, consider alternatives or strategies such as using data compression techniques.
Python Dictionary Gotchas
Mutable Keys
Unlike values, dictionary keys must be immutable. If you attempt to use a mutable data type as a key, you’ll encounter a TypeError. To avoid such issues, stick to using immutable keys like strings or tuples.
Order in Python 3.6 and Earlier
Before Python 3.7, dictionaries did not guarantee the order of elements. If order matters in your application, ensure you’re using Python 3.7 or later to benefit from ordered dictionaries.
Performance Comparison
When dealing with large datasets and frequent dictionary operations, it’s essential to consider performance implications. Here, we compare the performance of some common dictionary operations:
Access Time Complexity
- Accessing a Value by Key: O(1) – Dictionary keys are hashed, allowing for quick and direct access to values;
- Checking for Key Existence: O(1) – The in operator also operates in constant time.
Memory Usage
- Memory Overhead: Dictionaries have a memory overhead due to the storage of key-value pairs. This overhead can be significant for large dictionaries.
Performance Considerations
- Iteration Order (Python 3.7+): Python 3.7 onwards, dictionaries maintain insertion order. In earlier versions, dictionaries do not guarantee order;
- Hashing Algorithm: The performance of dictionary operations can be influenced by the quality of the hashing algorithm. Python’s built-in hash function is generally efficient, but custom objects may require custom hash functions for optimal performance.
Conclusion
In this extensive guide, we’ve delved into the world of Python dictionaries. You’ve learned what dictionaries are, how to create and initialize them, access and modify their elements, and utilize various methods. We’ve explored advanced concepts such as nested dictionaries and dictionary comprehensions and discussed common use cases and best practices.
By mastering the Python dictionary data structure, you’ve added a powerful tool to your programming arsenal, enabling you to tackle a wide range of tasks efficiently.
Now, it’s time to put your knowledge into practice and explore the endless possibilities that Python dictionaries offer.
FAQs
Python dictionaries are primarily used to store data in key-value pairs, allowing for efficient retrieval and manipulation of data based on unique keys.
Dictionary keys in Python must be of an immutable data type, such as strings, numbers, or tuples. This ensures that keys remain unique and consistent.
You can remove a key-value pair from a dictionary using the del statement or the pop() method, specifying the key you want to delete.
Python offers alternative data structures like lists, sets, and tuples, each with its own characteristics and use cases. The choice depends on the specific requirements of your program.
To check if a key exists in a dictionary, you can use the in keyword or the get() method. Using `get
Average Rating