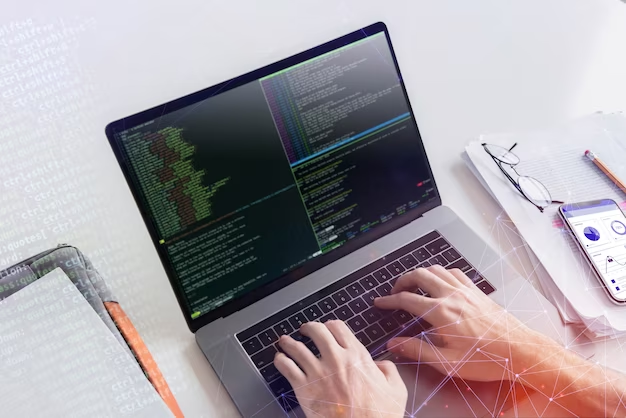
Managing Environment Variables in Python
Environment variables are fundamental variables that exist outside a program, operating at the system level. They are essential for configuring how a program behaves and securely storing sensitive information such as API keys or passwords. In this article, we will explore the world of Python environment variables, covering how to access, print, and manipulate them.
How to Get Environment Variables Using Python
In Python, you can access environment variables through the os module. The os.environ object provides a mapping of key-value pairs representing the process environment. To retrieve all environment variables, use the following code snippet:
import os # Get all environment variablesenv_vars = os.environ # Print environment variablesfor key, value in dict(env_vars).items(): print(f'{key} : {value}’) |
This code will display all your environment variables, including information about your system, user, and more.
If you wish to retrieve the value of a specific environment variable, use os.environ.get() as shown below:
import os # Get specific environment variableuser_var = os.environ.get(‘USER’) # Print the environment variableprint(user_var) |
This code retrieves the value associated with the ‘USER’ environment variable, which typically represents the current user’s name.
How to Set Environment Variables Using Python
You can also set environment variables using Python. The os. environ object allows you to insert key-value pairs into the environment, just like adding entries to a dictionary. Here’s how you can set an environment variable:
import os # Set a specific environment variableos.environ[‘TEST_PASSWORD’] = ‘12345’ # Get the specific environment variablepwd_var = os.environ.get(‘TEST_PASSWORD’) # Print the environment variableprint(pwd_var) |
In this example, we set the ‘TEST_PASSWORD’ environment variable to ‘12345’ and then retrieve and print its value.
Comparing Environment Variable Methods in Python
When working with environment variables in Python, you have several methods at your disposal for both accessing and modifying these crucial parameters. Let’s compare these methods to understand their strengths and use cases better.
Method | Accessing Environment Variables | Modifying Environment Variables | Use Cases |
---|---|---|---|
os.environ | Access all environment variables as a dictionary. | Not directly; changes apply only for the current session. | Retrieving all environment variables, inspecting the environment state. |
os.environ.get(‘VAR_NAME’) | Access a specific environment variable by name. | Not directly; changes apply only for the current session. | Retrieving specific environment variables by name. |
os.environ.setdefault(‘VAR_NAME’, ‘val’) | Access a specific environment variable by name, with a default. | Not directly; changes apply only for the current session. | Retrieving specific environment variables with a default value if not set. |
os.environ[‘VAR_NAME’] = ‘val’ | Not recommended; may raise errors if the variable is missing. | Set the value for a specific environment variable temporarily. | Temporarily setting environment variables during a Python session; not recommended for permanent changes. |
Choosing the Right Method
- For Retrieval: If you need to access environment variables for inspection purposes and don’t intend to modify them, use os.environ. It provides a dictionary-like interface to explore all environment variables and their values;
- For Specific Retrieval: When you want to fetch the value of a particular environment variable, use os.environ.get(‘VAR_NAME’). It’s a safer choice as it handles cases where the variable might not exist;
- Default Values: If you need to retrieve a specific variable but want to provide a default value when it’s not set, os.environ.setdefault(‘VAR_NAME’, ‘val’) is a handy option;
- Modifications (Temporary): While it’s possible to set environment variables directly using os.environ[‘VAR_NAME’] = ‘val’, this method is not recommended for permanent changes. It only affects the current Python session and may raise errors if the variable doesn’t exist.
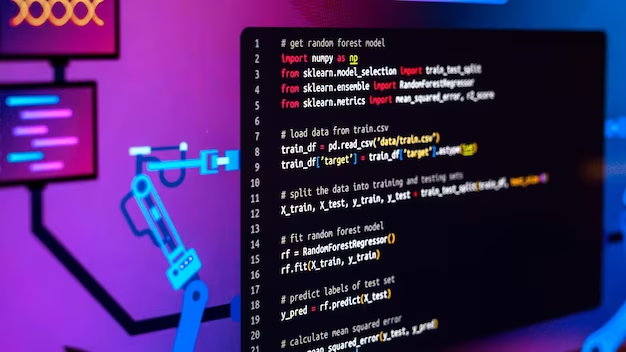
A Deep Dive into Environment Variables
Environment variables are essential for managing program behavior, security, and automation. They allow you to store sensitive data securely and configure your code for various environments. Whether you’re dealing with API keys, database credentials, or other configuration settings, understanding how to work with environment variables in Python is a valuable skill.
Exploring Advanced Techniques for Environment Variable Management
Managing environment variables is a crucial aspect of application development and system administration. In this section, we’ll delve into advanced techniques and best practices for handling environment variables effectively.
- Using a .env File
One common practice is to use a .env file to store environment variables. This file is typically kept separate from your codebase and contains key-value pairs. Tools like Python’s python-decouple or python-dotenv libraries make it easy to load these variables into your Python application.
Here’s an example of a .env file:
DATABASE_URL=postgres://username:password@localhost/dbnameSECRET_KEY=mysecretkeyDEBUG=True |
To use these variables in your Python code, you can do the following:
from decouple import config db_url = config(‘DATABASE_URL’)secret_key = config(‘SECRET_KEY’)debug = config(‘DEBUG’, default=False, cast=bool) |
This approach allows you to keep sensitive data separate from your code and easily switch between different configurations for development, staging, and production environments.
- Centralized Environment Variable Management
In larger projects or organizations, it’s essential to manage environment variables centrally. Tools like HashiCorp Vault or dedicated configuration management systems help securely manage sensitive information across multiple applications and environments.
These systems offer features like version control, access management, and auditing, making them suitable for enterprise-level projects.
- Automating Environment Variable Setup
When deploying applications to various environments (e.g., testing, staging, production), automating environment variable setup becomes crucial. Tools like Docker Compose, Kubernetes, and serverless platforms allow you to define environment variables as part of your deployment configuration.
For example, in a Docker Compose configuration file, you can specify environment variables like this:
version: ‘3’services: web: image: myapp environment: – DATABASE_URL=postgres://user:password@dbhost/dbname – DEBUG=True |
When you deploy your application using Docker Compose, these environment variables are automatically set within the container.
- Secrets Management
For storing and managing secrets (such as API keys, cryptographic keys, and passwords), consider using dedicated secret’s management solutions like HashiCorp Vault, AWS Secrets Manager, or Azure Key Vault. These services provide robust security and access control for sensitive data.
Video Explanation
In order to explain this topic in more detail, we have prepared a special video for you. Enjoy watching it!
Conclusion
Managing environment variables is a fundamental aspect of developing secure and configurable applications. By choosing the right method and adopting best practices, you can ensure that your Python applications handle sensitive data effectively, maintain portability across different environments, and adhere to security standards.
FAQ
Environment variables are external variables that exist at the operating system level and can affect the behavior of programs. In Python, they are crucial for storing sensitive information like API keys, database credentials, and configuration settings. Using environment variables enhances security and allows you to keep sensitive data separate from your codebase.
You can access environment variables in Python using the os module. The os.environ dictionary provides a convenient way to access all environment variables. You can also use os.environ.get(‘VAR_NAME’) to retrieve the value of a specific variable.
Using os.environ.get(‘VAR_NAME’) is safer because it handles cases where the specified variable might not exist. Directly accessing os.environ[‘VAR_NAME’] can raise errors if the variable is missing.
You can set environment variables for the current session in Python using os.environ[‘VAR_NAME’] = ‘value’. However, this method is not recommended for permanent changes. Changes made using this approach only apply during the current Python session.
Average Rating