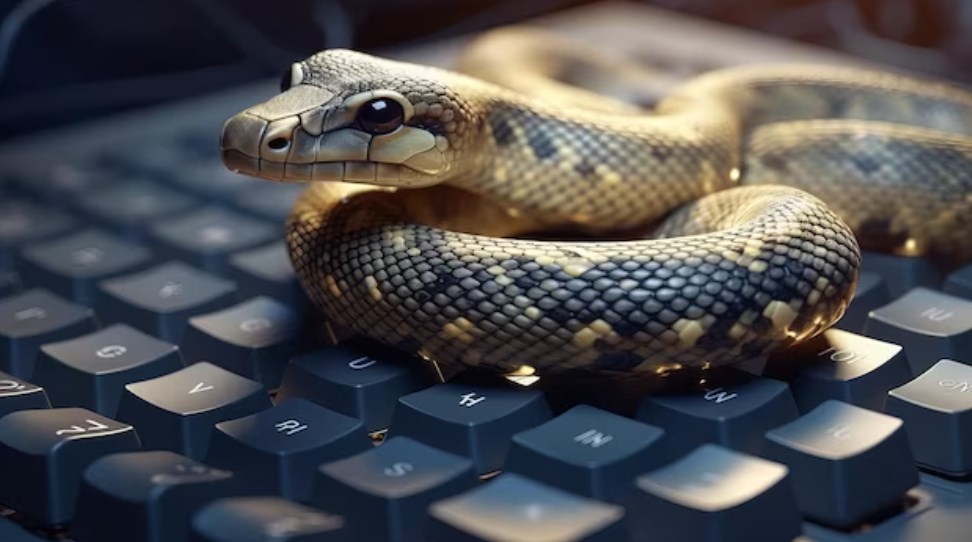
Image Retrieval from Web Sources Using Python
Working with visuals and automating their retrieval from various online platforms has become an increasingly prevalent subject in Python programming. The scope of these operations extends from straightforward visual manipulation to intricate endeavors like text analytics from visuals.
Typically, such media for training or testing algorithms can be either locally sourced or retrieved from web URLs.
This comprehensive tutorial delves deep into automating the download of visuals from web locations using Python. It offers insights into essential Python libraries, coding practices, and optimization techniques. Essential for novices and professionals alike, this guide aims to enhance the efficiency of your media-centric Python projects.
Retrieving Visuals from Web Addresses with Python
The first step in the journey to automate visual retrieval is understanding the required dependencies. One essential Python library is requests, which facilitates the sending and receiving of HTTP requests. To install this dependency, utilize the command prompt in Windows and execute:
pip install requests
Implementing Visual Download from Specific Web Addresses
To download a visual from a specific web address, one needs to identify the unique URL of the visual in question. Next, import the necessary Python packages and define the function to handle the retrieval. The function will generally take three arguments:
- web_address: This is the unique address where the visual is located;
- stored_name: This refers to the designated name under which the visual will be stored;
- http_headers: These are additional HTTP Headers that can be attached to the request.
import requests
def retrieve_visual(web_address, stored_name, http_headers):
Sending the HTTP Request and Receiving the Response
After defining the function, the next step is to send an HTTP GET request to the specified web address. This request returns a server’s response that can be evaluated.
response = requests.get(web_address, headers=http_headers)
Evaluating the Response and Storing the Visual
A successful HTTP request returns a Response code 200. Check for this code to ensure the retrieval was successful. Then proceed to store the visual locally.
if response.status_code == 200:
with open(stored_name, "wb") as visual_file:
visual_file.write(response.content)
else:
print("Failed to retrieve the visual. Error code:", response.status_code)
Executing the Code for Visual Retrieval
After preparing the function, all that remains is to provide the URL, the name under which the visual will be stored, and any necessary HTTP headers. Execute the code to complete the visual retrieval.
Sample Visuals for Testing
Various online resources provide sample visuals for testing retrieval scripts. For instance, a sample image might be located at a web address similar to this one: “https://example.com/sample.png”. Note the .png extension, indicating a specific image type. In this example, the image will be stored locally as sample_image.png.
Optimization Techniques
Optimizing the code for quicker and more efficient visual retrieval is often necessary, especially when dealing with large sets of data. One can consider asynchronous programming or multi-threading for concurrent visual downloads.
Handling Errors and Exceptions
Always code for error handling to ensure a smooth user experience. From issues like 404 Not Found to 403 Forbidden, having clear error messages and alternative actions can make a difference.
Advanced Download Features
For those who are looking to add more features, options like downloading visuals in different resolutions or formats can also be implemented. This often requires more complex scraping methods or APIs.
Automating the retrieval of visuals from web locations using Python is a skill that adds immense value to any data-centric project. This comprehensive tutorial provided an in-depth understanding of how to implement, optimize, and error-proof the visual retrieval process. With hands-on examples and advanced tips, it offers a complete toolkit for anyone looking to master this aspect of Python programming.
Utilizing HTTP Headers for Requests
The HTTP headers offer a vital role in automating the retrieval of visual media from the internet. Among various request headers, the User-Agent stands out as it allows web servers to recognize the software being utilized for the HTTP request. This is essentially a handshake between the client software and the web server.
import requests
def acquire_visual(web_location, local_name, request_headers):
http_response = requests.get(web_location, headers=request_headers)
if http_response.status_code == 200:
with open(local_name, "wb") as file_stream:
file_stream.write(http_response.content)
else:
print(http_response.status_code)
if __name__ == "__main__":
request_headers = {"User-Agent": "Chrome/51.0.2704.103"}
web_location = "https://pyshark.com/wp-content/uploads/2022/05/sampletext1-ocr-539x450.png"
local_name = "acquired_visual.png"
acquire_visual(web_location, local_name, request_headers)
Upon successful execution, a new file named acquired_visual.png should appear in the same directory where the script resides.
How to Set Up a Python Virtual Environment for Web Scraping
Setting up a Python virtual environment for web scraping can offer numerous benefits:
- Isolation from system-wide packages to avoid conflicts;
- Easier management of dependencies.
To set up a virtual environment, follow these steps:
- Install virtualenv via pip: pip install virtualenv;
- Create a new directory for your project;
- Inside this directory, run virtualenv venv to create a new virtual environment named venv;
- Activate the virtual environment;
- On Windows, use venv\Scripts\activate;
- On macOS and Linux, use source venv/bin/activate.
Best Practices for Structuring Your Web Scraping Project
The structure is the backbone of any robust, scalable project. Following best practices can save time and simplify debugging:
- Modular Design: Each distinct operation should be encapsulated within its own function;
- Error Handling: Implement robust error and exception handling mechanisms;
- Documentation: Comment the code clearly to explain the purpose of each function and what the variables represent;
- Configuration Files: Store configurations like URLs, file paths, and HTTP headers in separate configuration files.
Advanced Features – Implementing Download Rate Limiting
One advanced feature to consider is download rate limiting, especially when dealing with multiple downloads. This will help to not overwhelm the server and thus avoid getting blocked. This can be done by implementing sleep delays or by using Python libraries such as rate limiter.
Conclusion
Automating the acquisition of visual assets from online sources via Python is a multi-faceted topic with a broad range of applications. This tutorial aimed to provide a comprehensive overview, from the nitty-gritty details of making HTTP requests, to project structure and potential optimizations.
By applying these advanced techniques and following best practices, one can build scalable, efficient, and respectful web scraping applications. Understanding and implementing this skill could prove to be immensely beneficial, irrespective of whether one is a beginner looking to automate simple tasks or a seasoned developer looking to scale up complex projects.
Average Rating