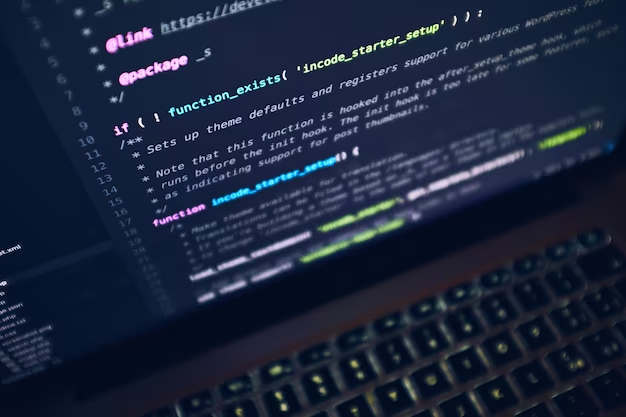
Mastering Spell Checking in Python
Spelling errors can sneak into our text, whether in code documentation, emails, or other text-based content. Python provides powerful tools to help us catch these errors and maintain accurate text. In this article, we’ll dive into the world of Python spell checking, learn how to verify words and sentences, and even build a simple spell checker program.
Checking Spelling with Python
To begin our journey into spell-checking with Python, we need to introduce a helpful library: textblob. Before we proceed, make sure you have it installed. If not, open your terminal or command prompt and use the following command:
pip install textblob |
Checking the Spelling of a Word
Let’s start by checking the spelling of a single word. We’ll use the textblob library to make this process easy.
Step 1
Import the Required Dependencies
from textblob import Word |
Step 2
Define a Word to Check Spelling
Choose a word with a potential spelling mistake and spell it incorrectly, like ‘apple’.
word = Word(‘appple’) |
Step 3
Check the Spelling of the Word
Now, let’s check the spelling of the word using the spellcheck() method.
result = word.spellcheck()print(result) |
You should see an output similar to this:
[(‘apple’, 1.0)] |
The result is a list of tuples, with the first element being the correct spelling suggestion (‘apple’ in this case), and the second element representing the confidence level.
Building a Program to Check Word Spelling
To create a program to check the spelling of a word, combine the above steps:
from textblob import Word def check_word_spelling(word): word = Word(word) result = word.spellcheck() if word == result[0][0]: print(f’Spelling of “{word}” is correct!’) else: print(f’Spelling of “{word}” is not correct!’) print(f’Correct spelling of “{word}”: “{result[0][0]}” (with {result[0][1]} confidence).’) check_word_spelling(‘appple’) |
This program will check the spelling of the word ‘appple’ and provide a suggestion along with confidence.
Checking the Spelling of a Sentence
To check the spelling of a sentence, we’ll build on the previous program. Unfortunately, we can’t check entire sentences at once, so we’ll split the sentence into words and spell-check each one.
- Step 1: Import the Required Dependencies
from textblob import Wordimport re |
- Step 2: Define a Sentence to Check Spelling
Create a sentence with spelling mistakes like ‘sentencee’ and ‘checkk’.
sentence = ‘This is a sentencee to checkk!’ |
- Step 3: Split the Sentence into Words
Split the sentence into individual words.
words = sentence.split() |
- Step 4: Convert Each Word to Lower Case
Convert each word to lowercase to improve spelling checking accuracy.
words = [word.lower() for word in words] |
- Step 5: Remove Punctuation Signs
Remove punctuation signs that can affect the spelling checker.
words = [re.sub(r'[^A-Za-z0-9]+’, ”, word) for word in words] |
- Step 6: Check the Spelling of Each Word in the Sentence
Use the check_word_spelling() function we created earlier to check the spelling of each word.
for word in words: check_word_spelling(word) |
The program will identify misspelled words and provide suggestions for correct spelling with confidence levels.
from textblob import Wordimport re def check_sentence_spelling(sentence): words = sentence.split() words = [word.lower() for word in words] words = [re.sub(r'[^A-Za-z0-9]+’, ”, word) for word in words] for word in words: check_word_spelling(word) check_sentence_spelling(‘This is a sentencee to checkk!’) |
This program will check the spelling of the sentence and provide suggestions for correct spelling along with confidence.
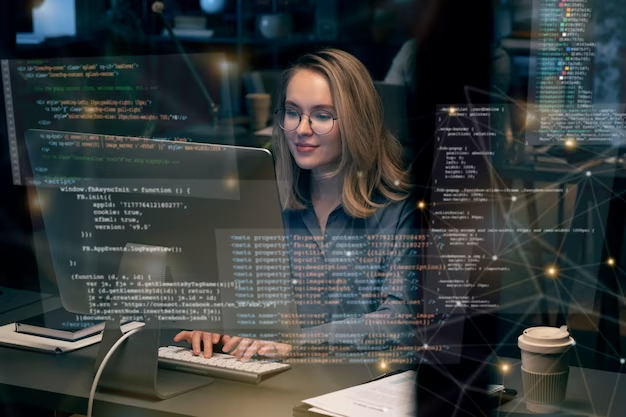
Comparison Table
Python Spell Check Libraries | Description | Use Cases | Popularity |
---|---|---|---|
TextBlob | A simple and intuitive library for text processing with built-in spell checking capabilities. | Blog posts, small projects | High |
pyspellchecker | A pure Python library that uses Peter Norvig’s spelling correction algorithm. | General-purpose spell checking | Moderate |
autocorrect | An ultra-lightweight library that offers spelling correction suggestions. | Quick and minimalistic spell checking | Low |
Gingerit | A Python wrapper for Ginger, an open-source grammar and spell checker. | Correcting grammar and spelling errors | Low |
These Python libraries offer various levels of complexity and customization for spell-checking tasks. Depending on your project’s requirements, you can choose the one that best suits your needs.
Video Explanation
In order to explain this topic in more detail, we have prepared a special video for you. Enjoy watching it!
Conclusion
In this article, we’ve explored how to perform spell checks on words and sentences using Python and the textblob library. Whether you’re writing code documentation or checking the accuracy of your text-based content, Python offers effective tools for ensuring correct spelling. If you have any questions or suggestions, feel free to leave a comment, and don’t forget to explore more Python programming tutorials.
FAQ
A Python spell checker is a software tool or library that helps identify and correct misspelled words in text. It can be used to improve the accuracy and readability of text-based applications.
The accuracy of Python spell checkers varies depending on the library and algorithm used. Some libraries, like TextBlob, offer high accuracy, while others may provide moderate accuracy. It’s essential to choose a spell checker that aligns with your specific requirements.
Yes, Python spell checkers can handle large documents, but their performance may vary. It’s advisable to test the spell checker’s performance on your specific document size and content to ensure optimal results.
Yes, many Python spell checkers support multiple languages and can be customized for non-English text. You can often specify the language you want to check during the spell-checking process.
Absolutely! Python spell checkers are versatile and can be integrated into web applications, automation scripts, and other text-based projects. They can help ensure that the text your applications generate or process is error-free.
Average Rating