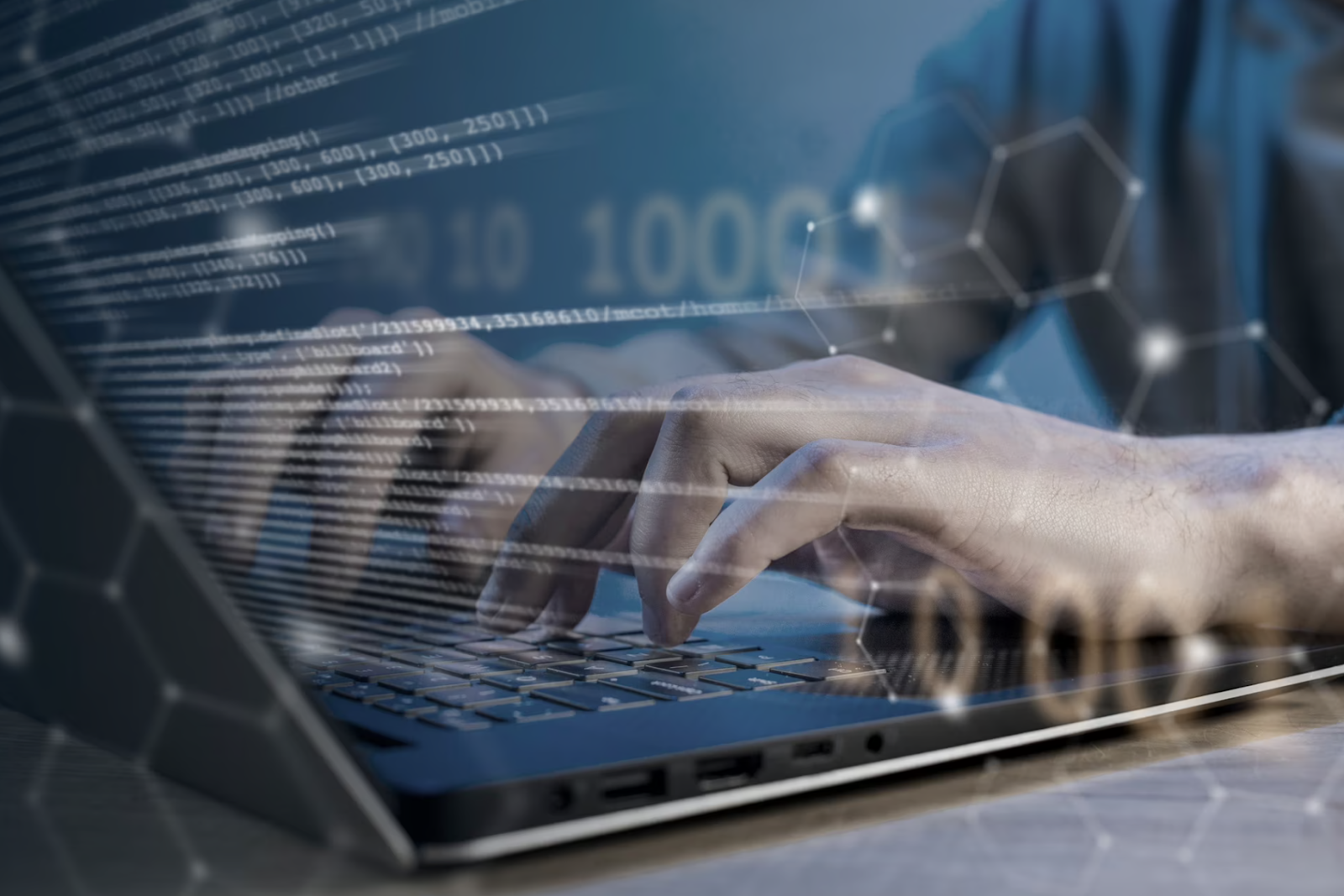
Mastering Trigonometric Functions in Python: A Guide
Trigonometric functions serve as the foundational building blocks for numerous mathematical and scientific applications. In the Python programming language, these indispensable functions are readily accessible, simplifying intricate calculations. Within this all-encompassing guide, we will delve deep into the realm of trigonometric functions in Python, beginning with essential principles and progressing to hands-on examples. By the end, you will possess the knowledge and expertise required to seamlessly incorporate these functions into your code, bolstering your computational capabilities significantly.
Trigonometry
Before we delve into specific trigonometric functions, let’s establish some essential trigonometric concepts that will serve as the foundation for our exploration.
Right Triangle
Trigonometry often begins with right triangles, where one angle is fixed at 90 degrees. The sides of a right triangle are known as the hypotenuse, adjacent side, and opposite side. Trigonometric functions relate these sides to the angles within the triangle.
Radian
While degrees are a common way to measure angles, radians offer a more natural approach for trigonometric functions. A radian measures the angle subtended at the center of a circle by an arc equal in length to the radius. It simplifies many trigonometric calculations, especially in calculus and advanced mathematics.
Sine Function
The sine function (sin) is one of the most fundamental trigonometric functions. It relates the angle of a right triangle to the ratio of the length of the side opposite the angle to the length of the hypotenuse. This relationship forms the basis for understanding periodic phenomena.
Sine Function Explained
In simpler terms, the sine of an angle tells you how high an object would reach when it moves along a circular path centered at the origin. Higher angles yield higher sine values, while lower angles produce lower values. This function is especially vital in wave analysis, such as modeling sound and light waves.
Sine Function Calculation Example
Let’s walk through a practical example of calculating the sine of an angle using Python. Imagine you have an angle of 30 degrees in a right triangle. To find the sine of this angle:
python
Copy code
import math angle_degrees = 30 angle_radians = math.radians(angle_degrees) # Convert degrees to radians sine_value = math.sin(angle_radians)
The math.radians() function converts the angle from degrees to radians, which is necessary for trigonometric functions in Python.
Sine Function sin() in Python
Python simplifies calculating the sine of an angle with the sin() function from the math library.
python
Copy code
import math angle_degrees = 30 sine_value = math.sin(math.radians(angle_degrees))
Inverse Sine Function
The inverse sine function (asin or arcsin) allows us to find the angle associated with a given sine value. It’s a powerful tool in solving problems involving angles, particularly when dealing with the inverse relationship of sine.
Inverse Sine Function Explained
The arcsin of a value yields the angle whose sine is the specified value. In other words, if you know the sine of an angle and want to find the angle itself, you would use the inverse sine function. It is commonly used in physics, engineering, and computer graphics to calculate angles.
Inverse Sine Function Calculation Example
Let’s consider an example where you want to find the angle whose sine is 0.5. To calculate this angle:
import math sine_value = 0.5 angle_radians = math.asin(sine_value) # Calculate the angle in radians angle_degrees = math.degrees(angle_radians) # Convert radians to degrees
The math.asin() function returns the angle in radians, so we convert it to degrees using math.degrees() for a more familiar measurement.
Inverse Sine Function asin() in Python
Python’s math library provides the asin() function for computing the inverse sine of a value.
import math sine_value = 0.5 angle_degrees = math.degrees(math.asin(sine_value))
Cosine Function
The cosine function (cos) complements the sine function and is another essential trigonometric function. It relates the angle of a right triangle to the ratio of the length of the adjacent side to the length of the hypotenuse.
Cosine Function Explained
In simpler terms, the cosine of an angle tells you how far an object moves horizontally when it travels along a circular path centered at the origin. Higher angles produce lower cosine values, and vice versa. Cosine plays a crucial role in various fields, including physics and engineering, where it helps calculate forces and vectors.
Cosine Function Calculation Example
Consider an example where you want to find the cosine of 60 degrees:
import math angle_degrees = 60 angle_radians = math.radians(angle_degrees) # Convert degrees to radians cosine_value = math.cos(angle_radians)
Here, we convert the angle from degrees to radians before calculating the cosine using the math.cos() function.
Inverse Cosine Function Explained
The arccos of a value yields the angle whose cosine is the specified value. It helps in situations where you have a known cosine value and need to find the corresponding angle.
Inverse Cosine Function Calculation Example
Let’s say you want to find the angle whose cosine is 0.5:
import math cosine_value = 0.5 angle_radians = math.acos(cosine_value) # Calculate the angle in radians angle_degrees = math.degrees(angle_radians) # Convert radians to degrees
In this example, we use the math.acos() function to calculate the angle in radians and then convert it to degrees.
Inverse Cosine Function acos() in Python:
Python’s math library provides the acos() function for computing the inverse cosine of a value.
import math cosine_value = 0.5 angle_degrees = math.degrees(math.acos(cosine_value))
Tangent Function
The tangent function (tan) is another essential trigonometric function that relates the angle of a right triangle to the ratio of the length of the side opposite the angle to the length of the side adjacent to it.
Tangent Function Explained
In simple terms, the tangent of an angle tells you the slope of a line in the Cartesian coordinate system. It’s a crucial function in fields such as physics and engineering, where it helps calculate slopes and rates of change.
Tangent Function Calculation Example
Consider an example where you want to find the tangent of 45 degrees:
import math angle_degrees = 45 angle_radians = math.radians(angle_degrees) # Convert degrees to radians tangent_value = math.tan(angle_radians)
We convert the angle from degrees to radians before calculating the tangent using the math.tan() function.
Tangent Function tan() in Python
Python’s math library simplifies calculating the tangent of an angle with the tan() function.
import math angle_degrees = 45 tangent_value = math.tan(math.radians(angle_degrees))
Inverse Tangent Function
The inverse tangent function (atan or arctan) helps you find the angle associated with a given tangent value. It’s widely used in solving problems involving angles and slopes.
Inverse Tangent Function Explained
The arctan of a value yields the angle whose tangent is the specified value. It is particularly useful in scenarios where you have a known tangent value and need to determine the corresponding angle.
Inverse Tangent Function Calculation Example
Let’s say you want to find the angle whose tangent is 1:
import math tangent_value = 1 angle_radians = math.atan(tangent_value) # Calculate the angle in radians angle_degrees = math.degrees(angle_radians) # Convert radians to degrees
Here, we use the math.atan() function to calculate the angle in radians and then convert it to degrees.
Inverse Tangent Function atan() in Python
Python’s math library provides the atan() function for computing the inverse tangent of a value.
import math tangent_value = 1 angle_degrees = math.degrees(math.atan(tangent_value))
Conclusion
Mastering trigonometric functions in Python is a valuable skill that opens doors to a wide range of applications in mathematics, science, and engineering. Whether you’re calculating angles, distances, or slopes, Python’s built-in trigonometric functions and libraries make complex calculations accessible. However, it’s essential to use these functions responsibly and ethically, ensuring their application aligns with legal and ethical standards. With the knowledge gained from this guide, you’re well-equipped to tackle real-world problems that involve trigonometric calculations and leverage Python’s capabilities to solve complex mathematical and scientific challenges.
Average Rating