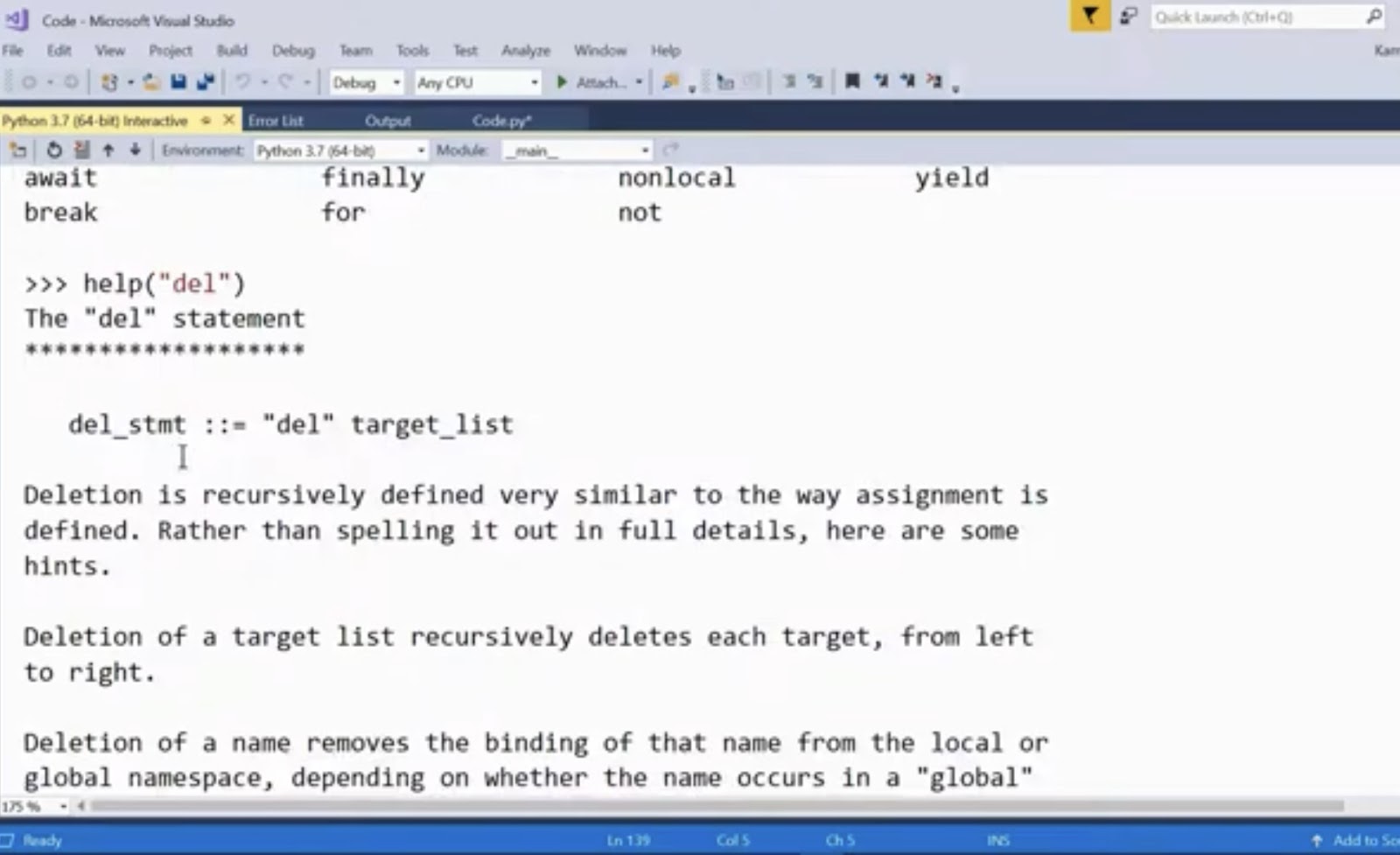
Mastering the Python help() Function for Assistance
In Python, individuals frequently encounter new modules, functions, classes, or objects that they haven’t previously used and for which they haven’t yet reviewed the documentation. Rather than sifting through documentation websites searching for a particular function or class, Python offers the “help()” function to swiftly access this information. The Python “help()” function serves the purpose of displaying documentation for a specified module, function, class, or object. Here’s a breakdown of how to use it.
Accessing Documentation Using the Interactive Help Utility
Users can initiate the Python “help()” function without any arguments to activate an interactive prompt with the help utility. This utility can be employed to access documentation for any Python object. To illustrate, here’s how to start the interactive help utility:
- Upon invoking “help()” without any arguments, Python graciously opens the doors to its vast knowledge repository. This interactive help utility becomes a guiding companion for programmers navigating the Python landscape. It greets users with a welcoming message, providing essential information for newcomers, including references to tutorials and external resources;
- The interactive help utility is akin to a treasure map, allowing users to delve into Python’s intricacies with ease. It facilitates explorations by enabling users to enter the name of any module, keyword, or topic, subsequently delivering a wealth of knowledge at their fingertips. This feature transforms the learning process into an interactive and immersive journey, making Python accessible to learners of all levels;
- Furthermore, the utility extends its functionality by offering lists of available modules, keywords, symbols, and topics. This feature promotes discoverability, aiding users in identifying the tools and resources they need to fulfill their programming objectives.
In essence, the interactive help utility is a testament to Python’s commitment to user-friendly design. It empowers users to interact with Python’s extensive ecosystem confidently, fostering a deeper understanding of its capabilities and nuances. With “help()” as a trusty guide, Python enthusiasts embark on a journey of continuous learning and discovery within the realm of this versatile programming language.
```python
# Initiate the help utility
help()
```
Upon running this, a help utility will appear in the terminal, such as:
Welcome to Python 3.7’s help utility!
If this is your first time using Python, you should definitely check out
the tutorial on the Internet at https://docs.python.org/3.7/tutorial/.
To get a list of available modules, keywords, symbols, or topics, type
“modules”, “keywords”, “symbols”, or “topics”. Each module also comes
with a one-line summary of what it does; to list the modules whose name
or summary contain a given string such as “spam”, type “modules spam”.
help>
“`
Subsequently, within the interactive help utility, users can look up documentation on Python objects like this:
```python
# Finding documentation for Python map() function
help> map
```
This will yield the function’s documentation:
```
Help on class map in module builtins:
class map(object)
map(func, *iterables) --> map object
Make an iterator that computes the function using arguments from each of the iterables. Stops when the shortest iterable is exhausted.
```
As demonstrated, the documentation provides a comprehensive description of the function, its methods, and docstrings.
Accessing Object Documentation Directly Using “help()”
Users can also access Python object documentation directly in one step, bypassing the interactive help utility. This is achieved by utilizing the Python “help()” function with a Python object passed as an argument in the following format:
```python
# Accessing Python map() function documentation directly
help(map)
```
This will yield the same documentation as earlier, displaying the function’s details.
Accessing User-Defined Function Documentation Using “help()”
The Python “help()” function is not limited to built-in functions; it can also display information about user-defined functions. To illustrate, after creating a simple function like this:
```python
# Define a function
def add(x, y):
```
This function adds two given integer arguments
Parameters:
x : integer
y : integer
Output:
val: integer
```
val = x + y
return val
```
You can call “help()” on this user-defined function:
```python
# Finding documentation for the user-defined function "add"
help(add)
```
This will display documentation from the function’s docstring, including its description, input parameters, and return value.
Conclusion
In conclusion, the Python “help()” function proves to be an invaluable tool for both newcomers and experienced developers in the Python programming ecosystem. Its multifaceted utility extends to a wide array of scenarios, simplifying the process of accessing essential documentation.
- The interactive help utility, initiated simply by invoking “help()” without any arguments, serves as a dynamic portal to the Python world. It offers a gateway for users to swiftly retrieve comprehensive information about modules, functions, classes, or objects. This interactive feature greatly expedites the learning curve for those new to Python and provides a convenient reference point for experienced programmers;
- Moreover, the ability to access documentation directly using “help()” ensures efficiency in documentation retrieval. Users can bypass the interactive utility and retrieve precise details about Python objects with ease, streamlining their development workflow;
- A notable advantage of the “help()” function is its flexibility in handling user-defined functions. It not only accommodates built-in Python functions but also offers insights into functions crafted by developers themselves. This promotes best practices by encouraging the inclusion of docstrings, enhancing code maintainability, and facilitating collaboration among developers.
In essence, the Python “help()” function exemplifies Python’s commitment to user-friendliness and accessibility. It empowers programmers to harness the full potential of Python libraries and modules while promoting good coding practices. Whether you are a Python novice exploring the basics or a seasoned coder delving into complex libraries, “help()” is a versatile companion that simplifies the journey, fosters understanding, and enhances the overall Python programming experience. Its utility extends beyond lines of code, contributing to a thriving Python community and ecosystem.
Average Rating